How to Find Odd Even Numbers in JavaScript?
The article introduces three methods for finding odd and even numbers in JavaScript: the modulus operator, bitwise operations, and array filtering.
Do you know how to find odd and even numbers in JavaScript? This might seem like a simple task, but correctly distinguishing between odd and even numbers is crucial when writing code. This article will introduce you to several methods for finding odd and even numbers in JavaScript, providing simple and understandable code examples.
1.Definition of Odd and Even Numbers
First, let's clarify the concepts of odd and even numbers. In mathematics, an odd number is an integer that cannot be divided evenly by 2, while an even number is an integer that can be divided evenly by 2. For example, 3 is odd because it cannot be divided by 2 evenly, whereas 4 is even because it can be divided by 2 evenly.
2.Using the Modulus Operator to Determine Odd and Even Numbers
The modulus operator (%) in JavaScript can help us quickly determine whether a number is odd or even. The modulus operator returns the remainder of a division operation. If a number divided by 2 leaves a remainder of 0, then it is even; otherwise, it is odd. Here's a simple example code:
function isEvenOrOdd(num) {
if (num % 2 === 0) {
console.log(num + " is even");
} else {
console.log(num + " is odd");
}
}
isEvenOrOdd(5); // Output: "5 is odd"
isEvenOrOdd(8); // Output: "8 is even"
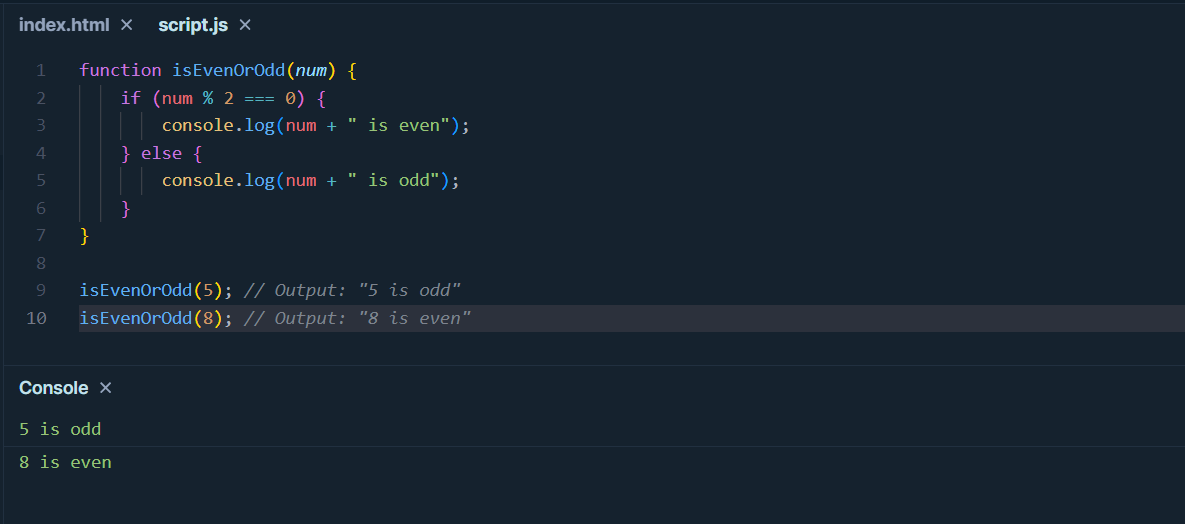
3.Using Bitwise Operations to Determine Odd and Even Numbers
Apart from the modulus operator, we can also use bitwise operations to determine odd and even numbers. Bitwise operations are a more efficient method. We can use the bitwise AND operator (&) to check if the least significant bit of a number's binary representation is 1; if it is, the number is odd, otherwise, it is even. Here's the corresponding code example:
function isEvenOrOdd(num) {
if (num & 1) {
console.log(num + " is odd");
} else {
console.log(num + " is even");
}
}
isEvenOrOdd(5); // Output: "5 is odd"
isEvenOrOdd(8); // Output: "8 is even"
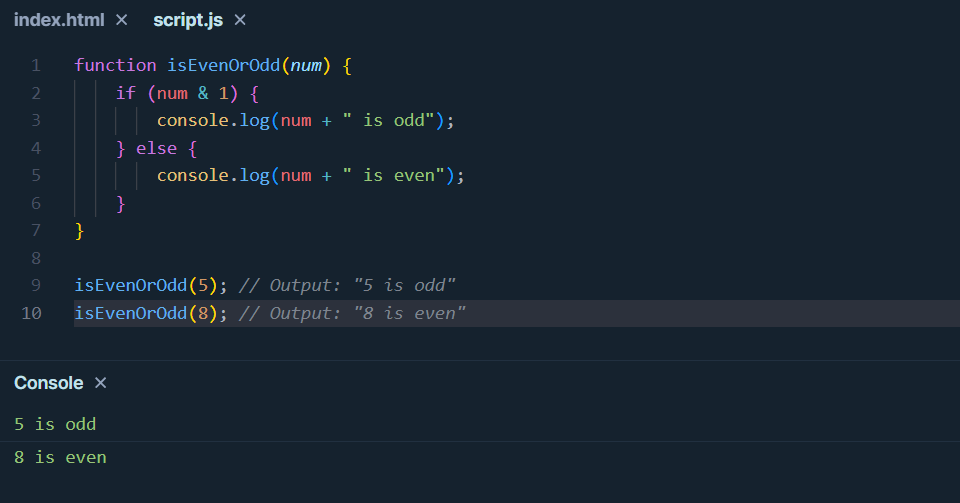
4.Using Array Filtering Methods to Filter Odd and Even Numbers
If you need to find all odd or even numbers in an array, you can use the array filtering methods in JavaScript. Here's a simple example:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evens = numbers.filter(num => num % 2 === 0);
const odds = numbers.filter(num => num % 2 !== 0);
console.log("Evens:", evens); // Output: [2, 4, 6, 8, 10]
console.log("Odds:", odds); // Output: [1, 3, 5, 7, 9]
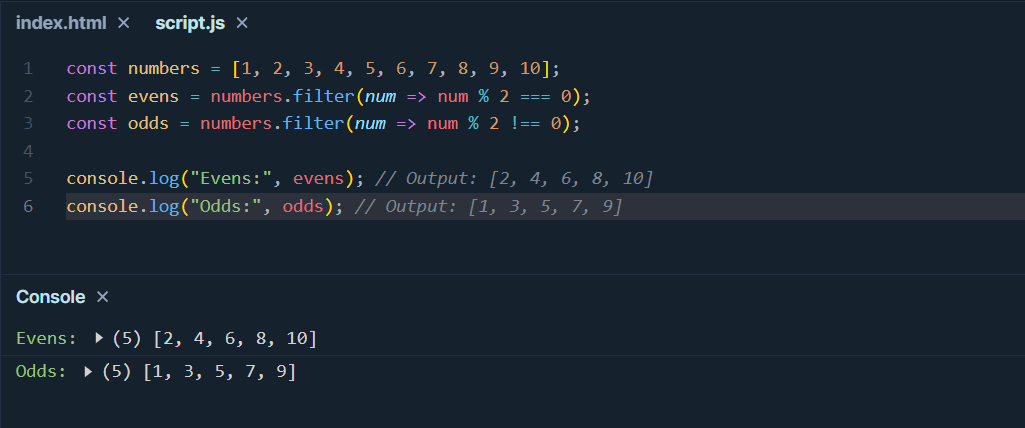
5.Performance Comparison and Best Practices
When choosing a method, performance should be considered. Generally, bitwise operations are the fastest method, followed by the modulus operator. However, in actual development, the most suitable method should be chosen based on the specific situation.
Summary
This article introduced three methods for finding odd and even numbers in JavaScript: the modulus operator, bitwise operations, and array filtering. The modulus operator checks whether the remainder is 0, bitwise operations examine whether the least significant bit is 1, and array filtering selects based on the remainder. In terms of performance, bitwise operations are the fastest, followed by the modulus operator. Choose the most suitable method according to your needs.
Reference Links:
- JavaScript.info: Bitwise Operators
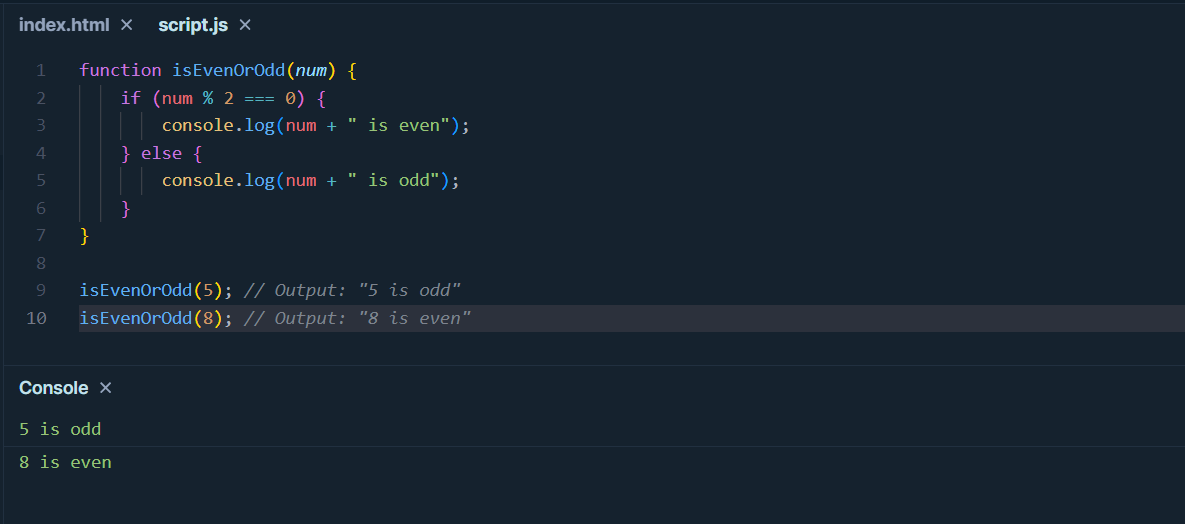
Learn more: