How to Get Last Day of Month in JavaScript?
This article introduces methods to get the last day of the current month in JavaScript, including using native JavaScript, the Moment.js library, and the Day.js library.
When developing web applications, manipulating dates is often necessary, and one common requirement is to obtain the last day of the current month. This is useful in many scenarios such as generating monthly reports, handling recurring tasks, and more. However, date manipulation in JavaScript is not always straightforward. Therefore, in this article, we will delve into why and how to get the last day of the current month in JavaScript, providing several methods to achieve this goal.
Why Do We Need to Get the Last Day of the Current Month?
Obtaining the last day of the current month is a common requirement when dealing with date ranges. For example, if you need to generate a monthly report, you may want to ensure that the report includes all data for the current month, not just up to the current date. Additionally, when dealing with recurring tasks, it is sometimes necessary to know the last day of the current month to adjust schedules.
Methods to Get the Last Day of the Current Month in JavaScript
Vanilla JavaScript
One common method is to use JavaScript's built-in Date object to get the current date and calculate the last day based on the current month. Here's a basic example code:
var currentDate = new Date();
var nextMonthFirstDay = new Date(currentDate.getFullYear(), currentDate.getMonth() + 1, 1);
var lastDayOfMonth = new Date(nextMonthFirstDay - 1);
console.log("The last day of the current month is: " + lastDayOfMonth.getDate());
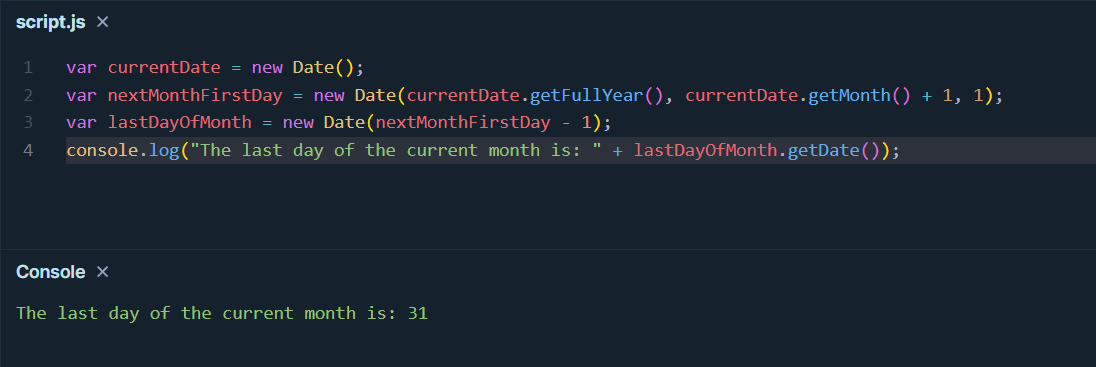
In addition to the basic method, you can also use existing date manipulation libraries or frameworks to simplify the process of getting the last day of the current month. Two popular choices are Moment.js and Day.js.
Moment.js Library
Moment.js is a powerful and flexible date manipulation library that provides many convenient methods for handling dates and times. Here's an example code using Moment.js to get the last day of the current month:
var lastDayOfMonth = moment().endOf('month');
console.log("The last day of the current month is: " + lastDayOfMonth.date());
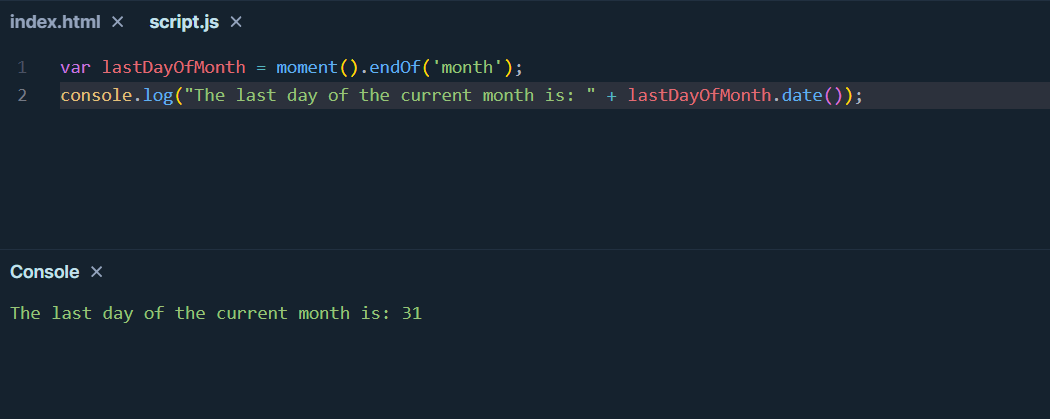
You need to include Moment.js in your project, referencing the import method described in the Moment.js official documentation.
Day.js Library
Day.js is a lightweight date manipulation library with an API design similar to Moment.js but lighter. Here's an example code using Day.js to get the last day of the current month:
var lastDayOfMonth = dayjs().endOf('month');
console.log("The last day of the current month is: " + lastDayOfMonth.date());
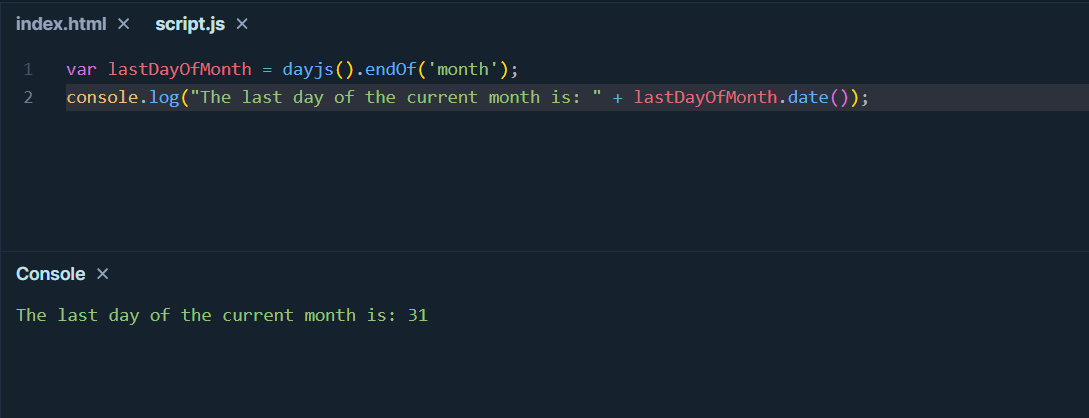
You need to include Day.js in your project, referencing the import method described in the Day.js official documentation.
Considerations
When dealing with dates, it's also important to consider other factors, such as spanning across years, different time zones and localization settings, as well as error handling and edge cases. Make sure to take these factors into account in your actual application to ensure that your code works correctly in various scenarios.
Conclusion
In this article, we explored several methods to get the last day of the current month in JavaScript, including basic JavaScript methods and utilizing date manipulation libraries like Moment.js and Day.js. Choose the method that best fits your project requirements, and remember to keep learning and exploring JavaScript's date manipulation techniques.
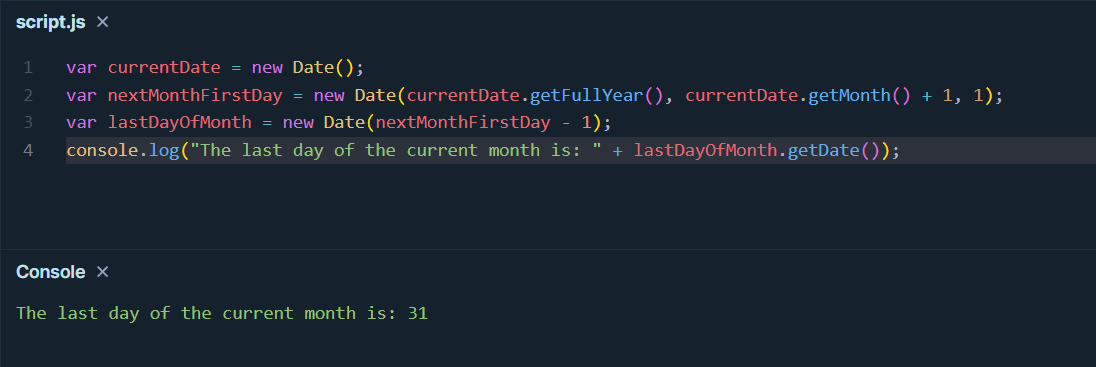
Learn more: