How to format dates in JavaScript?
This article discusses how to format dates in JavaScript, using both native built-in JavaScript methods and third-party libraries such as moment.js.
When writing JavaScript applications, date formatting is a common task. Today, we will explore how to format dates in JavaScript, and I will demonstrate these methods through code examples.
Introduction to Date Objects in JavaScript
The date object in JavaScript is Date
, which allows us to get and set the date and time. Here are some common methods:
// Create a Date object
let now = new Date();
// Get year, month, and date
let year = now.getFullYear();
let month = now.getMonth() + 1; // Note: months start from 0
let date = now.getDate();
// Get hours, minutes, and seconds
let hours = now.getHours();
let minutes = now.getMinutes();
let seconds = now.getSeconds();
The need for date formatting
Date formatting is necessary in various contexts, such as displaying dates on web pages, logging, data processing, etc. We need to format dates flexibly according to different needs.
Basic date formatting methods
Basic date formatting can be achieved through string concatenation or using built-in methods as follows:
// Get current date and time
let now = new Date();
// Get year, month, date, hours, minutes, seconds
let year = now.getFullYear();
let month = now.getMonth() + 1;
let date = now.getDate();
let hours = now.getHours();
let minutes = now.getMinutes();
let seconds = now.getSeconds();
// Format as yyyy-mm-dd
let formattedDate = `${year}-${month < 10 ? '0' + month : month}-${date < 10 ? '0' + date : date}`;
// Format as hh:mm:ss
let formattedTime = `${hours < 10 ? '0' + hours : hours}:${minutes < 10 ? '0' + minutes : minutes}:${seconds < 10 ? '0' + seconds : seconds}`;
console.log("Current date:", formattedDate);
console.log("Current time:", formattedTime);
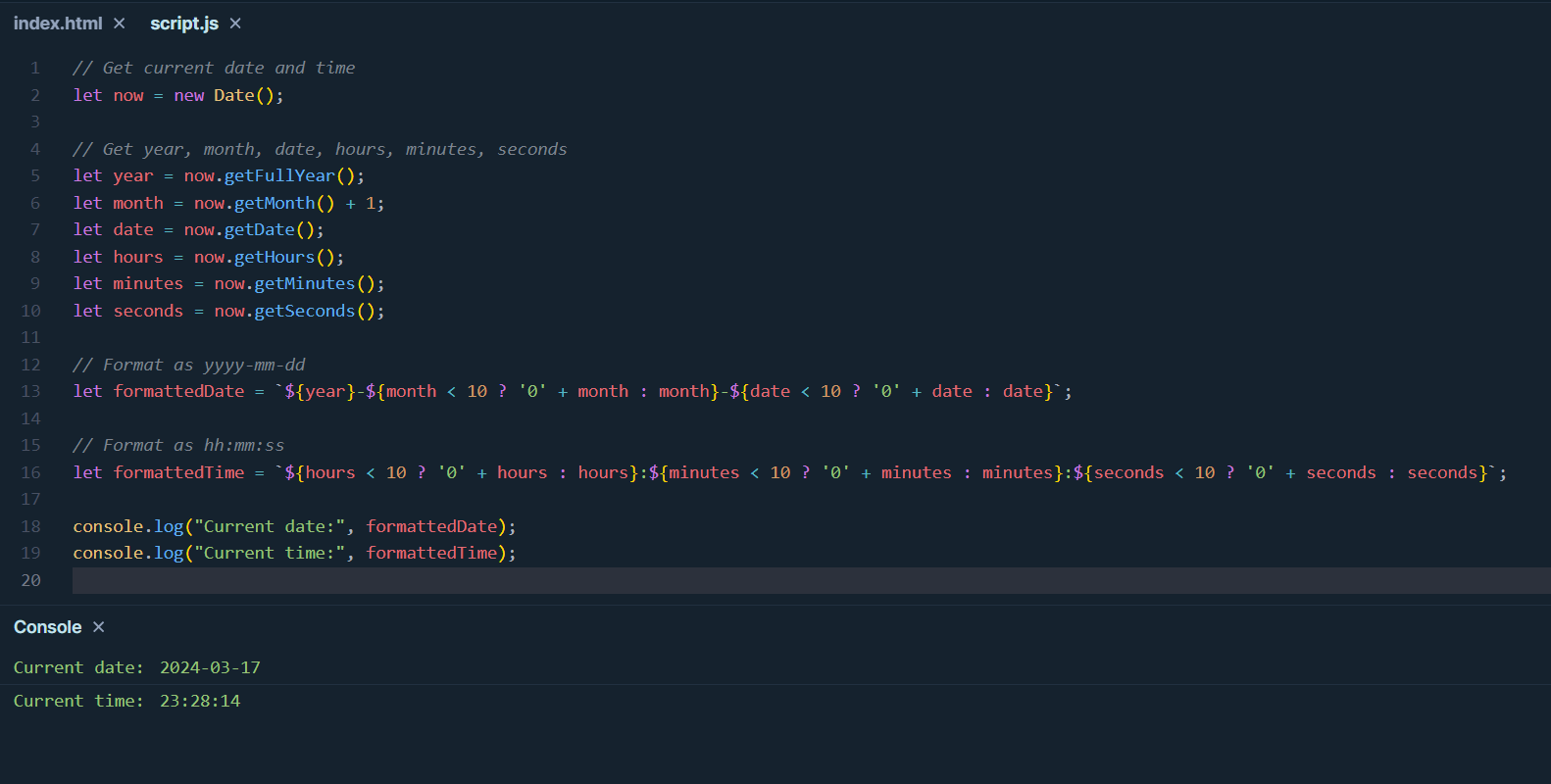
Advanced tips for date formatting
When dealing with complex date formatting requirements, we can use third-party libraries such as moment.js . Here is a simple usage example:
<!-- Include moment.js -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js"></script>
<script>
let now = new Date();
// Format date using moment.js
let formattedDate = moment(now).format('YYYY-MM-DD');
let formattedTime = moment(now).format('HH:mm:ss');
console.log(formattedDate);
console.log(formattedTime);
</script>
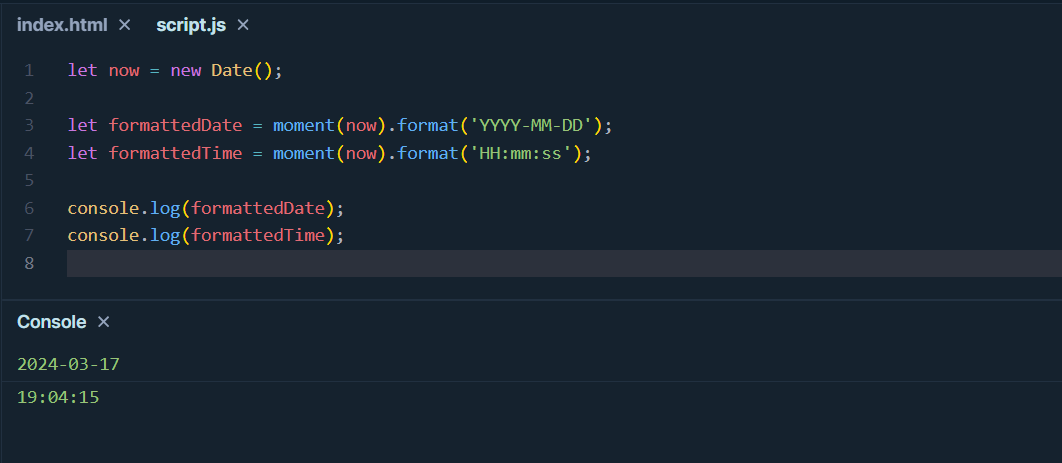
Summary
Date formatting is a fundamental skill in JavaScript development. By mastering the basic methods and utilizing third-party libraries, we can easily meet various date formatting needs.
Reference link:
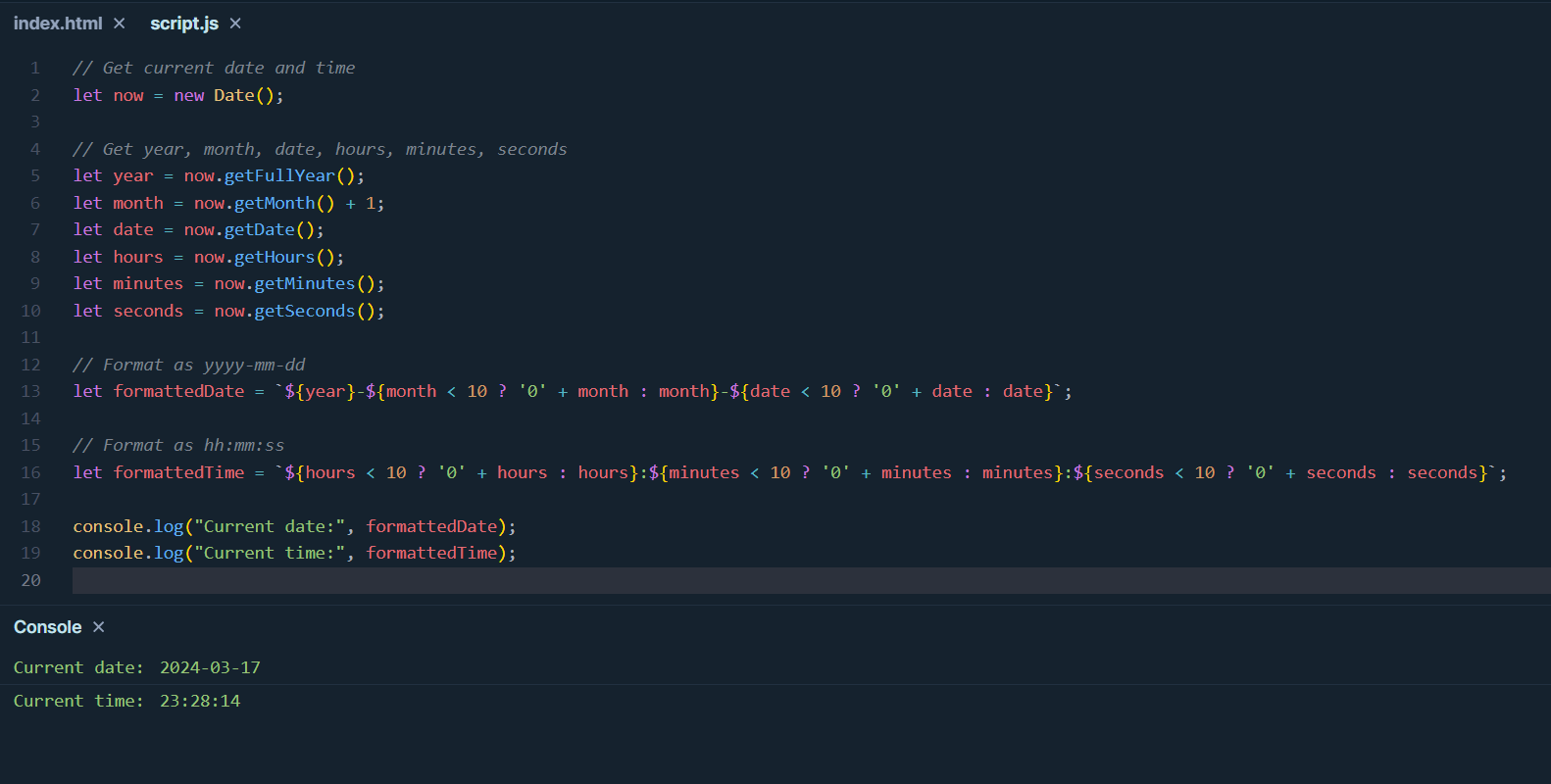
Learn more: