How to Add Variables in Regex JavaScript?
One is to use the constructor of the RegExp object, by passing in string variables to create dynamic regular expressions. The other is to combine regular expression literals with variables, directly inserting variables into the regular expression literal to achieve dynamic matching.
In this article, we explore how to incorporate variables into regular expressions in JavaScript. Regular expressions are powerful tools for handling pattern matching and searching within strings, and adding variables can make our regular expressions more flexible and intelligent. In this piece, we delve into how to accomplish this and discuss some common use cases.
Regular Expression Basics
First, let's review the basics of regular expressions. In JavaScript, regular expressions consist of metacharacters, quantifiers, character classes, and more. Metacharacters such as "^", "$", ".", etc., represent the beginning, end, or any character in a string to be matched. Quantifiers like "*", "+", "?", etc., specify the quantity of characters to match, while character classes, denoted by square brackets "[]", match any character from a specified set.
Methods to Add Variables in Regular Expressions
So, how do we add variables to regular expressions in JavaScript? There are two common methods. One is to use the constructor of the RegExp object, passing in string variables to create dynamic regular expressions. The other is to combine regular expression literals with variables, directly inserting variables into the regular expression literal to achieve dynamic matching.
1.Using the Constructor of the RegExp Object
With the constructor of the RegExp object, we can dynamically create regular expressions, thus adding variables flexibly.
Example:
// Create a string containing a variable
var pattern = "apple";
var flags = "gi"; // g: global search, i: case insensitive
// Create a dynamic regular expression using the RegExp constructor
var regex = new RegExp(pattern, flags);
// Test the match
var text = "I have an apple and an APPLE.";
var matches = text.match(regex);
console.log(matches); // ["apple", "APPLE"]
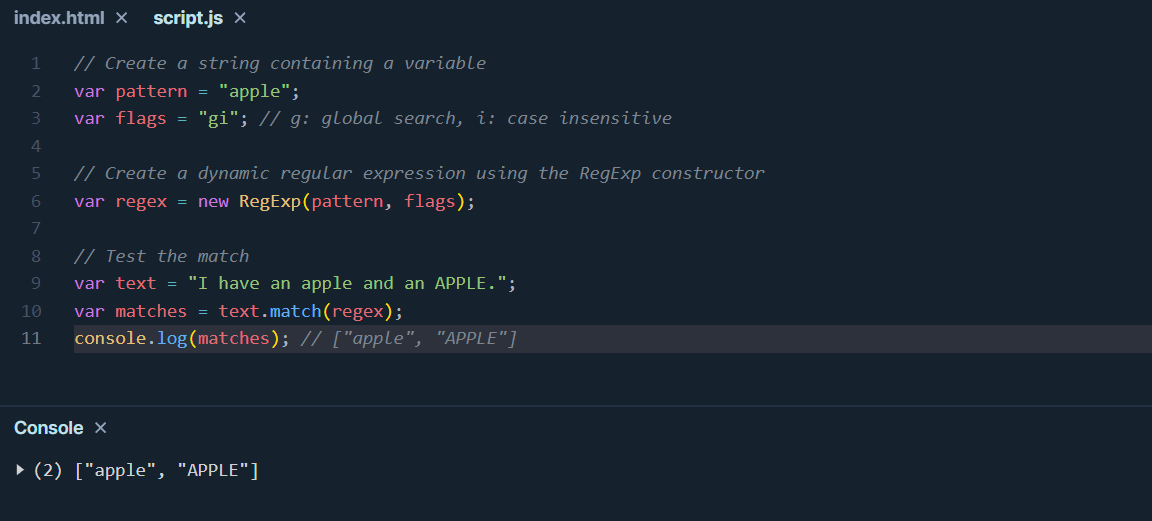
2.Combining Regular Expression Literals with Variables
We can also directly insert variables into regular expression literals to achieve dynamic matching.
Example:
// Create a string containing a variable
var fruit = "apple";
var regex = new RegExp(fruit, "gi"); // g: global search, i: case insensitive
// Combine regular expression literal with variable
var text = "I have an apple and an APPLE.";
var matches = text.match(regex);
console.log(matches); // ["apple", "APPLE"]
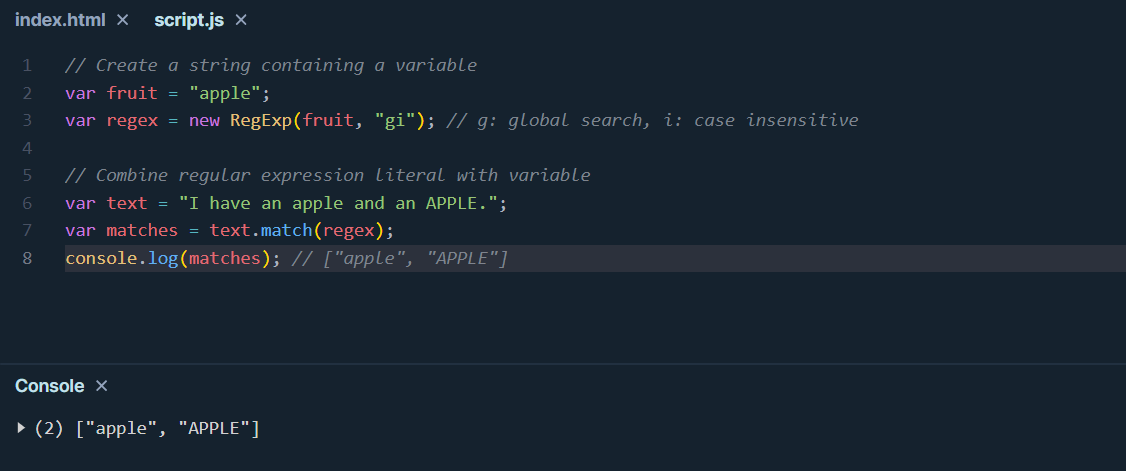
Both of these methods enable dynamic regular expressions in JavaScript, allowing us to match content within strings based on the value of variables. The choice between the two depends on personal preference and project requirements.
Considerations
When using regular expressions, we need to be careful of special characters that may cause errors, so extra caution is required when constructing dynamic regular expressions. Additionally, we should consider edge cases and boundary conditions to ensure our regular expressions work correctly. It's best practice to use the constructor of the RegExp object for handling dynamic regular expressions, as it offers more flexibility and safety.
Conclusion
In summary, this article has discussed how to add variables to regular expressions in JavaScript and explored some common use cases. Understanding how to incorporate variables into regular expressions can help us better handle string matching and searching, improving the flexibility and maintainability of our code.
References:
- Mozilla Developer Network (MDN) :https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_Expressions
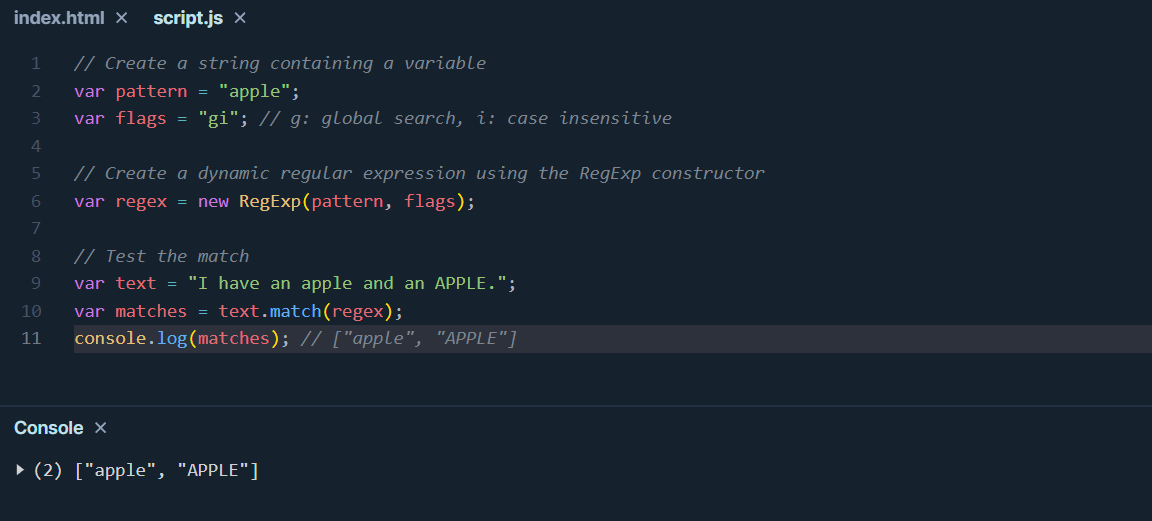
Learn more:
- How to Disable Back Button in Browser Using JavaScript?
- How to disable selection options using JavaScript?
Learn more: