What are Postman tests and how to write?
Postman's Tests are automatically executed scripts used to validate if request responses meet expectations. They are written in JavaScript and offer predefined assertion functions to check various aspects of responses.
Tests in Postman are scripts that are automatically executed after sending a request to verify if the response meets the expected criteria. These tests are written in JavaScript and come with a set of predefined assertion functions to check various aspects of the response, such as status codes, response body content, and response time.
Tests scripts can be written individually for each request or shared at the Collection or Folder level in Postman. The steps to write Tests in Postman are as follows:
1.Create a new request in Postman
Open Postman, create or open a request, then select the "Tests" tab in the top navigation bar of the request editor. In the "Tests" tab, you can write JavaScript code to validate the response.
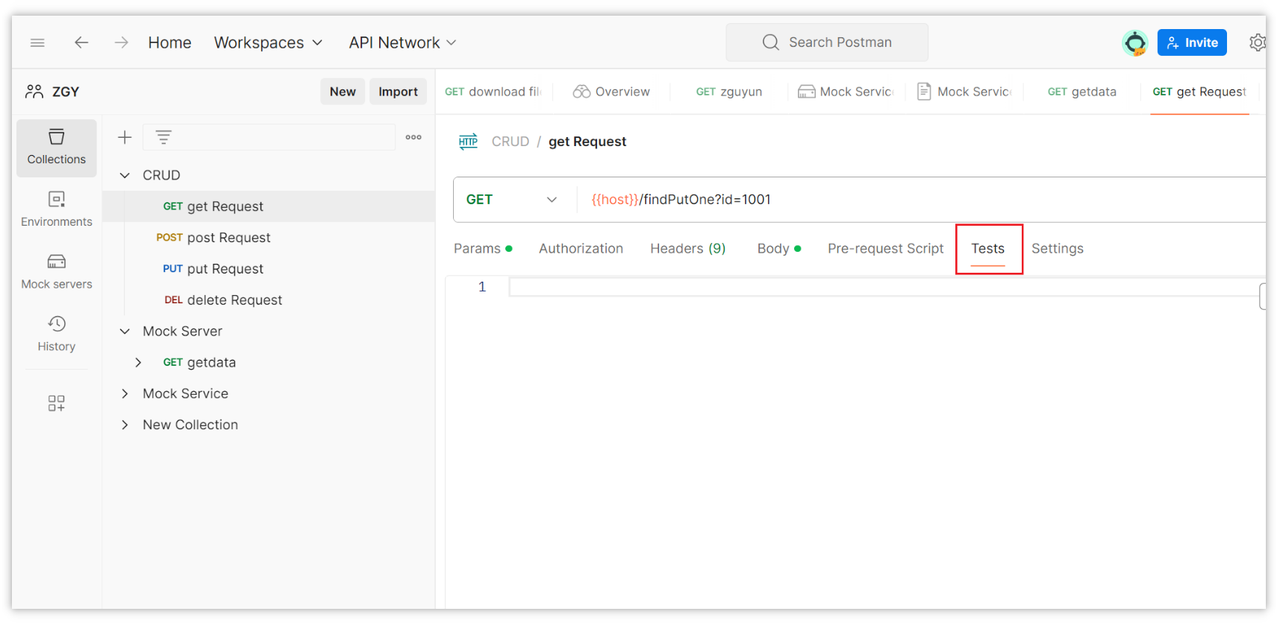
2.Write the script in Tests
Predefined assertion functions can be used to check different aspects of the response, such as pm.response.to.have.status()
to verify the status code, or pm.response.to.have.jsonBody()
to check JSON response bodies. Below is a simple example demonstrating how to write a basic Tests script to validate the response status code:
// Check if the response status code is 200
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
3.Send the request and verify the results
After writing tests, click the "Send" button to send the request. Tests will be automatically executed, and the results will be displayed in the "Test Results" panel.
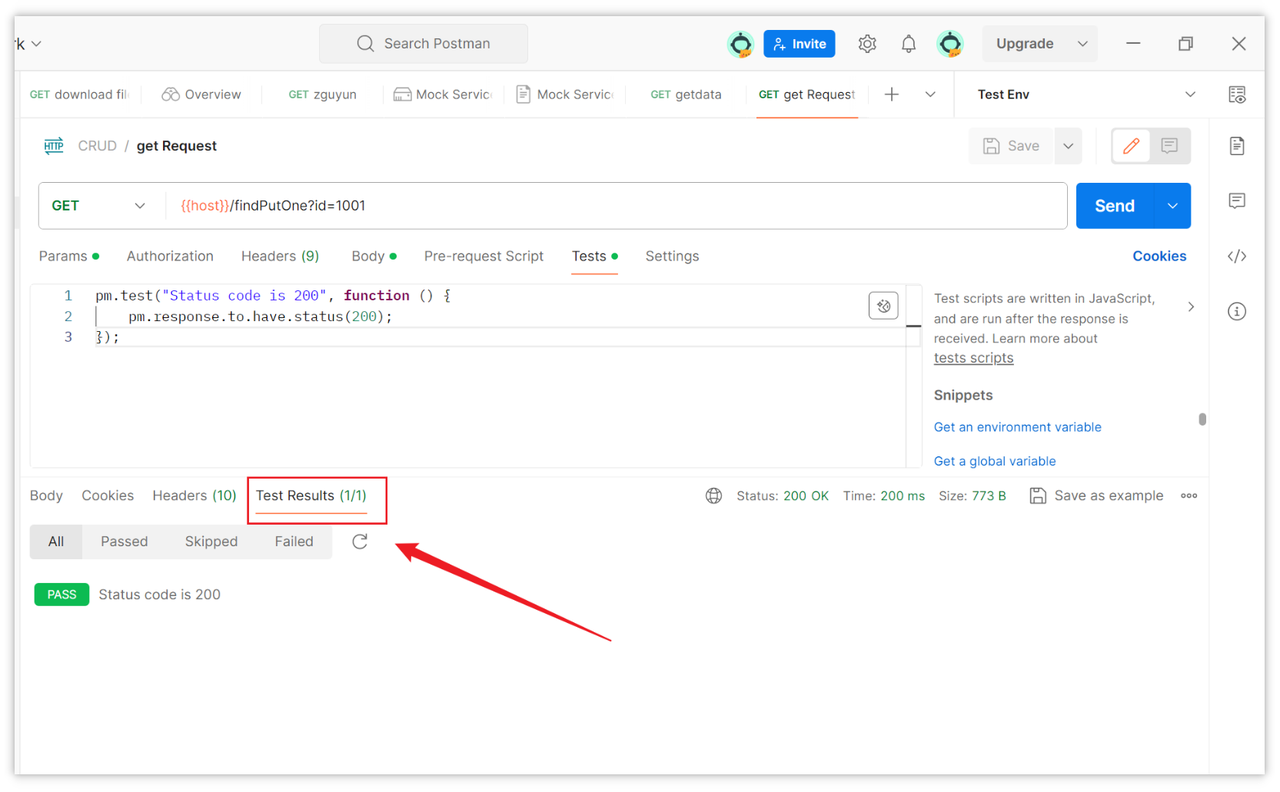
Apart from verifying status codes, there are many other common Tests scripts for validating various aspects of the request. Here are some examples:
- Check if specific fields are present in the response body:
pm.test("Response body contains expected field", function () {
var jsonData = pm.response.json();
pm.expect(jsonData).to.have.property('field_name');
});
- Verify if the response time is within the expected range:
pm.test("Response time is less than 500ms", function () {
pm.expect(pm.response.responseTime).to.be.below(500);
});
- Ensure that the response header contains specific information:
pm.test("Response header contains expected value", function () {
pm.response.to.have.header('header_name');
});
- Check if specific fields in the response body have expected values:
pm.test("Response body field has expected value", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.field_name).to.eql('expected_value');
});
- Validate if the response body conforms to a specific JSON structure:
pm.test("Response body has expected JSON structure", function () {
pm.response.to.have.jsonBody('json_schema');
});
- Verify if the length of an array in the response body matches the expected length:
pm.test("Response body array has expected length", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.array_name).to.have.lengthOf(3);
});
These are some common examples of Tests scripts. You can write custom Tests scripts according to your specific testing requirements. It is recommended to refer to the Postman official documentation for more information on predefined assertion functions and testing techniques.
Summary
Postman's Tests are automatically executed scripts used to validate if request responses meet expectations. They are written in JavaScript and offer predefined assertion functions to check various aspects of responses. Tests can be written for each request or shared at the Collection/Folder level. Besides verifying status codes, Tests can check response bodies, response times, response headers, field values, etc.
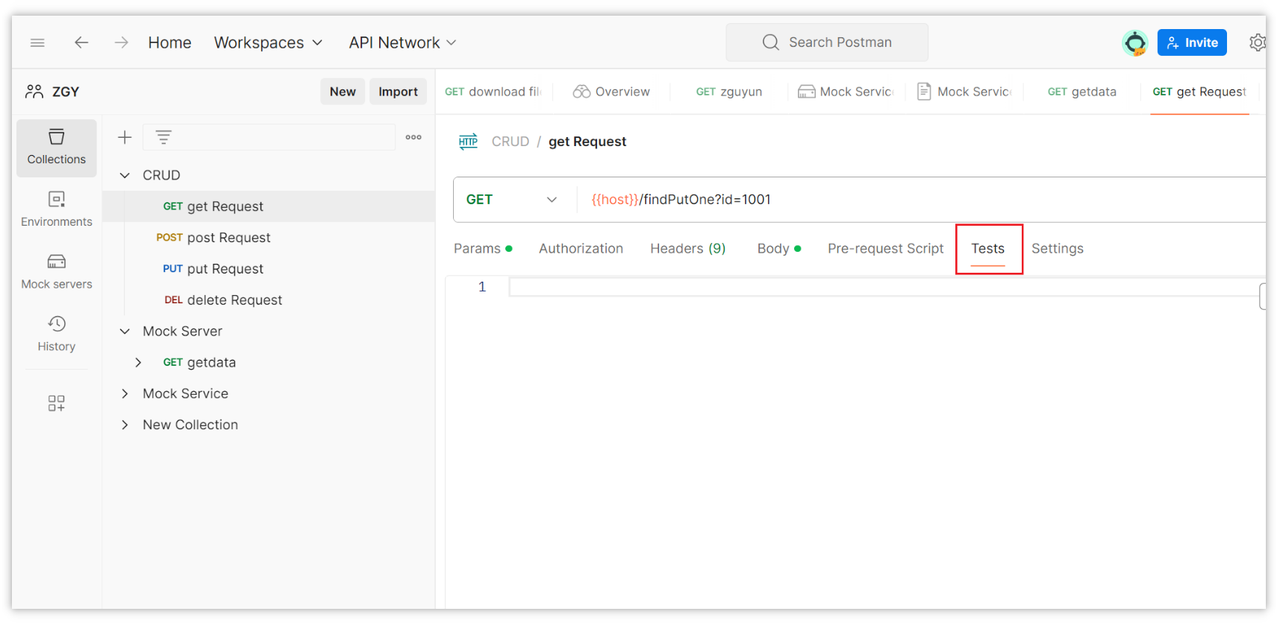
Reference:
Learn more: