How to Execute cURL Commands in Python?
Through this article, we have learned several methods for executing cURL commands in Python, including using the os or subprocess module, the requests library, and the pycurl library.
cURL is a powerful command-line tool used for communicating with various network protocols, including HTTP, FTP, and more. Combining cURL with Python offers more flexibility and control, whether for automated testing, data collection, or web development. This article introduces various methods for executing cURL commands in Python.
cURL Basics
The structure of cURL commands is simple and intuitive, with common parameters such as URL, request method, and data. For example, to retrieve the content of a web page, you can use curl
https://zguyun.com
. Through this simple example, we can understand the basic usage of cURL.
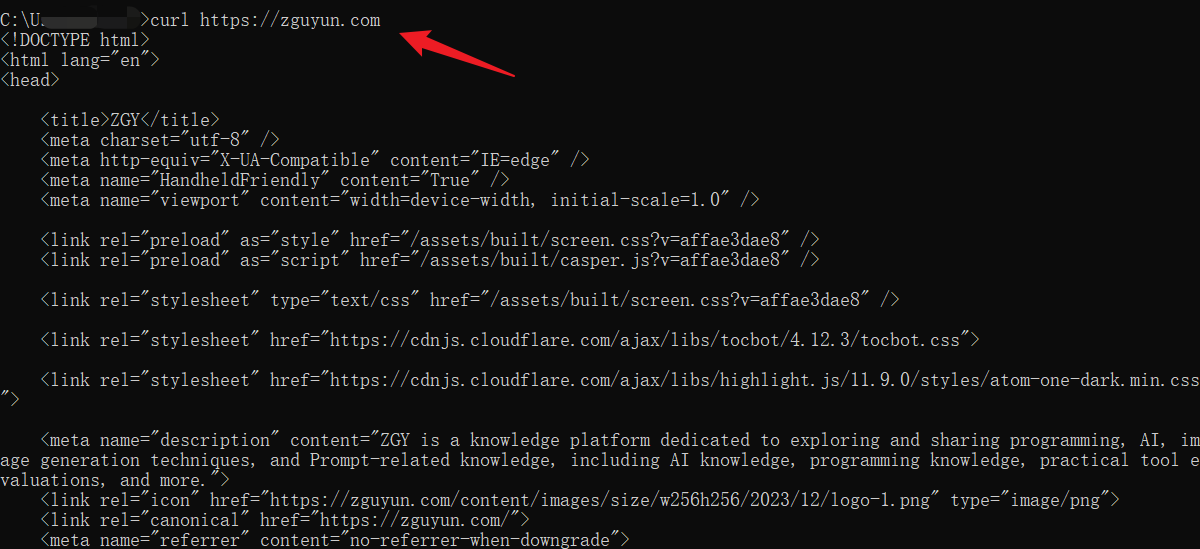
Methods for Executing cURL Commands in Python
1.Using the os or subprocess Module
Below are simple example codes demonstrating how to use the os module and the subprocess module to execute cURL GET requests, with brief explanations for each line of code:
import subprocess
import os
# Using the subprocess module to execute cURL GET request
subprocess.run(['curl', '-X', 'GET', 'https://zguyun.com'])
# Using the os module to execute cURL GET request
os.system("curl https://zguyun.com")
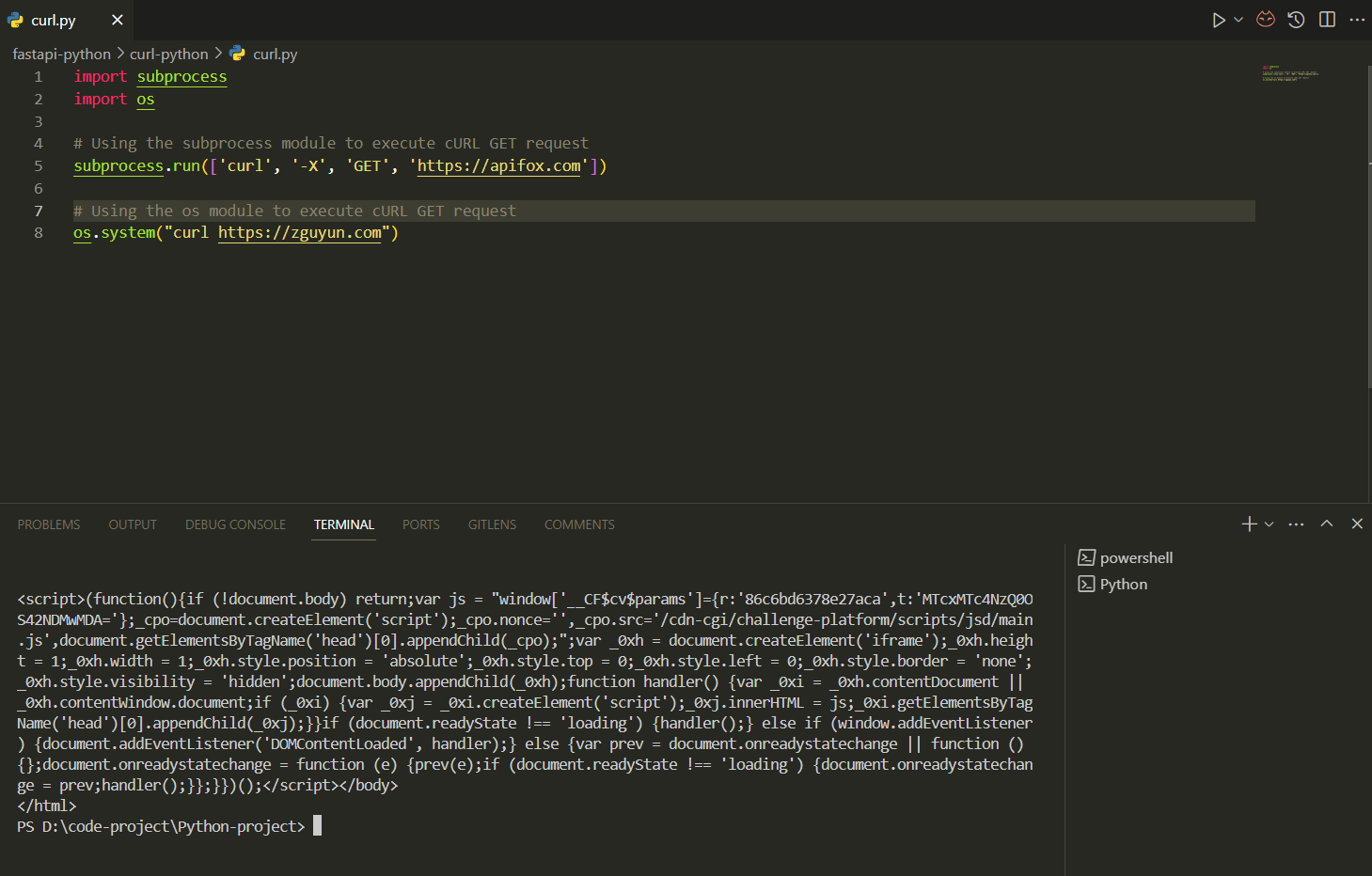
Executing cURL GET request using the subprocess module:
- We use the
subprocess.run()
function to execute the cURL command. - The arguments passed to
subprocess.run()
is a list containing the cURL command and its parameters. - This line of code will execute the
curl -X GET https://zguyun.com
command in the command line, sending a GET request tohttps://zguyun.com
.
Executing cURL GET request using the os module:
- We use the
os.system()
function to execute the cURL command. - The argument passed to
os.system()
function is a string, which is the complete cURL command. - This line of code will also execute the
curl https://zguyun.com
command in the command line, sending a GET request tohttps://zguyun.com
.
2.Using the requests Library
The requests library is an advanced tool in Python for handling HTTP requests. It provides a richer API and better exception handling mechanism, making HTTP communication more convenient. Sending cURL commands using requests library is as follows:
import requests
# Sending a GET request
response = requests.get("https://zguyun.com")
response.encoding = 'utf-8'
print(response.text)
# Sending a POST request
url = "https://example.com/post_endpoint"
data = {"key": "value"} # Data for POST request
response = requests.post(url, data=data)
response.encoding = 'utf-8'
print(response.text)
# Setting headers and sending a GET request
url = "https://example.com/get_endpoint"
headers = {"token": "a9igh9382t209gj2904g-234gh42fvfw"} # Setting headers
response = requests.get(url, headers=headers)
response.encoding = 'utf-8'
print(response.text)
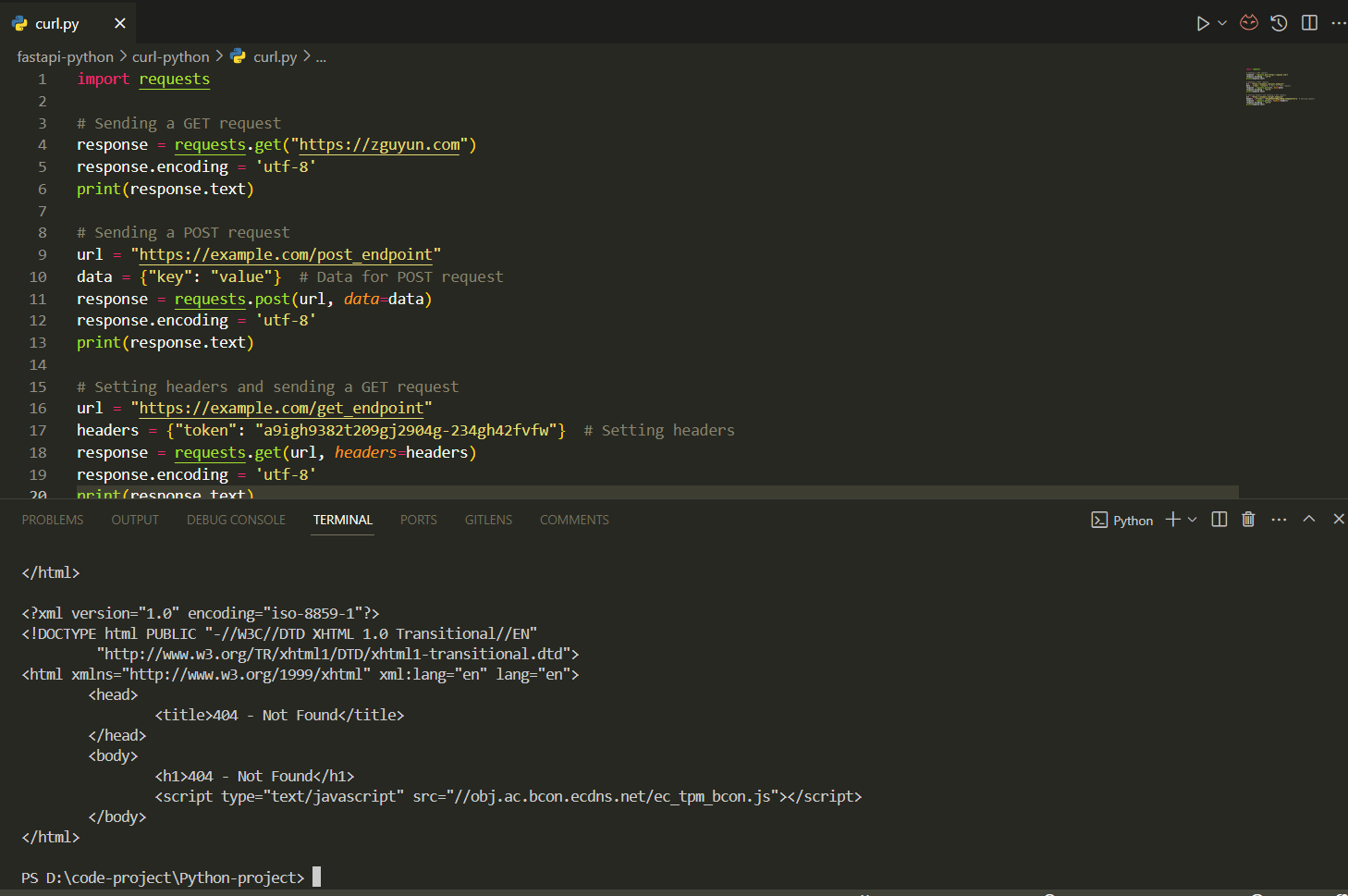
3.Using the pycurl Library
The pycurl library is the Python binding for libcurl, providing similar functionality to the cURL command-line tool. By installing pycurl and using it, we can execute cURL commands in Python scripts.
Installation command:
pip install pycurl
Example usage of pycurl library:
import pycurl
from io import BytesIO
buffer = BytesIO()
c = pycurl.Curl()
c.setopt(c.URL, "https://zguyun.com")
c.setopt(c.WRITEDATA, buffer)
c.perform()
c.close()
body = buffer.getvalue()
print(body.decode('utf-8'))
Conclusion
Through this article, we have learned several methods for executing cURL commands in Python, including using the os or subprocess module, the requests library, and the pycurl library. Each method has its advantages and disadvantages, and we can choose the appropriate method based on specific requirements.
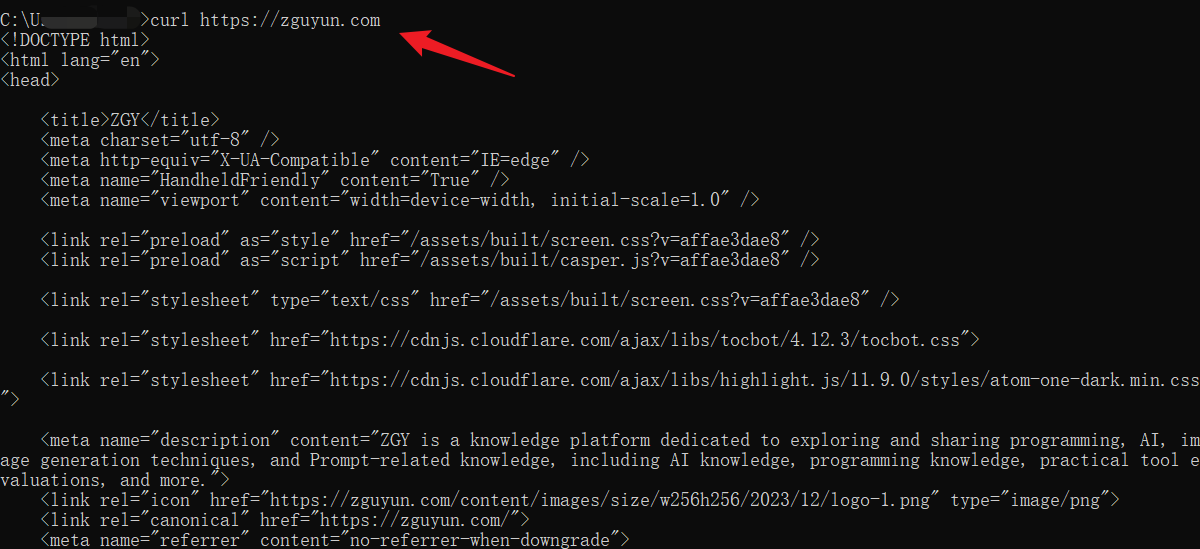
Learn more: