How to convert date to epoch time in JavaScript?
This article introduces several methods to convert dates into Epoch time in JavaScript, namely using the getTime() method and timestamp functions.
Today, let's talk about a common yet crucial topic in JavaScript - how to convert dates into Epoch time (also known as Unix timestamps). You might have encountered situations where it's necessary to pass time information between different systems or applications, and Epoch time serves as a convenient standard for that. In this article, I'll explain in detail what Epoch time is, why we need it, and demonstrate several methods to convert dates into Epoch time in JavaScript.
What is Epoch time?
Epoch time, also known as Unix timestamp, refers to the number of seconds elapsed since the beginning of Coordinated Universal Time (UTC) on January 1, 1970. This particular moment is termed as the Unix epoch and is widely accepted as the reference point for time, hence allowing representation of any arbitrary point in time conveniently.
Why do we need Epoch time?
There are several benefits to converting dates into Epoch time:
- When transmitting time information between different systems, Epoch time serves as a universal standard.
- It facilitates comparisons and calculations of time, especially in programming.
- Epoch time is a widely used time representation across most programming languages and operating systems, ensuring interoperability.
Methods to convert dates into Epoch time in JavaScript
Method 1: Using the getTime() method
The Date object in JavaScript has a method named getTime(), which returns the number of milliseconds elapsed since January 1, 1970, 00:00:00 UTC till now. We can use it to obtain the Epoch time of the current date-time.
const now = new Date();
const epochTime = Math.floor(now.getTime() / 1000); // Convert milliseconds to seconds
console.log(epochTime);
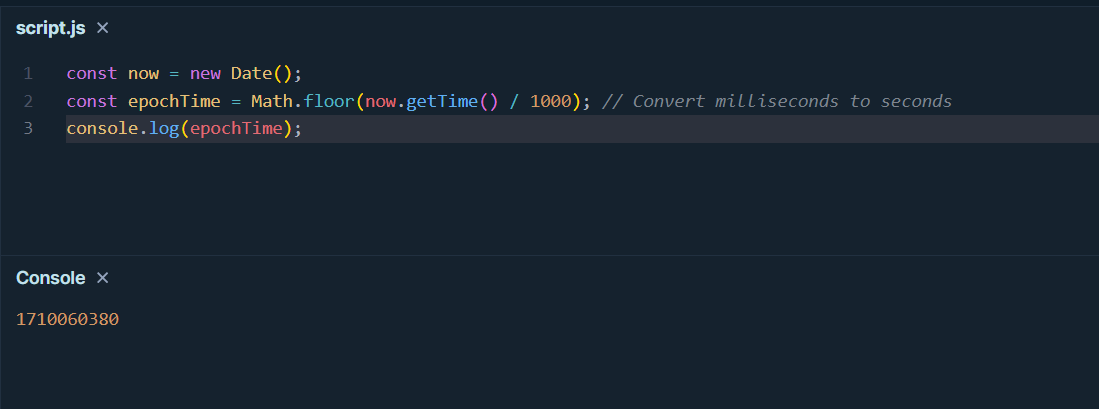
Method 2: Using timestamp functions
Apart from the getTime() method, we can also use some timestamp functions to obtain Epoch time, such as Date.now().
const epochTime = Math.floor(Date.now() / 1000); // Convert milliseconds to seconds
console.log(epochTime);
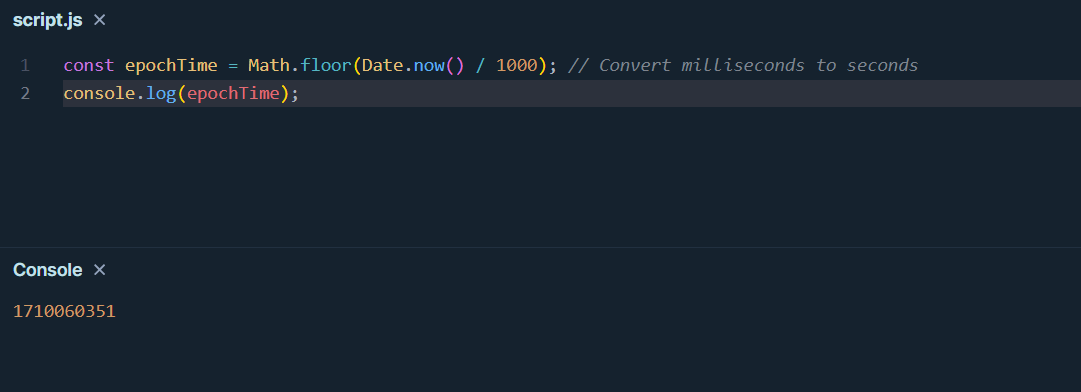
Considerations
- Timezone issues: Note that Date objects in JavaScript are processed on the client-side and may be influenced by the user's local timezone settings.
- Handling edge cases: When dealing with date conversions, consider some edge cases such as leap seconds, timezone changes, etc.
- Exception handling: In real-world applications, ensure proper exception handling to maintain code stability and reliability.
Conclusion
This article has introduced several methods to convert dates into Epoch time in JavaScript, and discussed the importance and applications of Epoch time. Whether it's for time comparison, calculating time differences, or transmitting time across systems, Epoch time serves as a very convenient standard.
Reference:
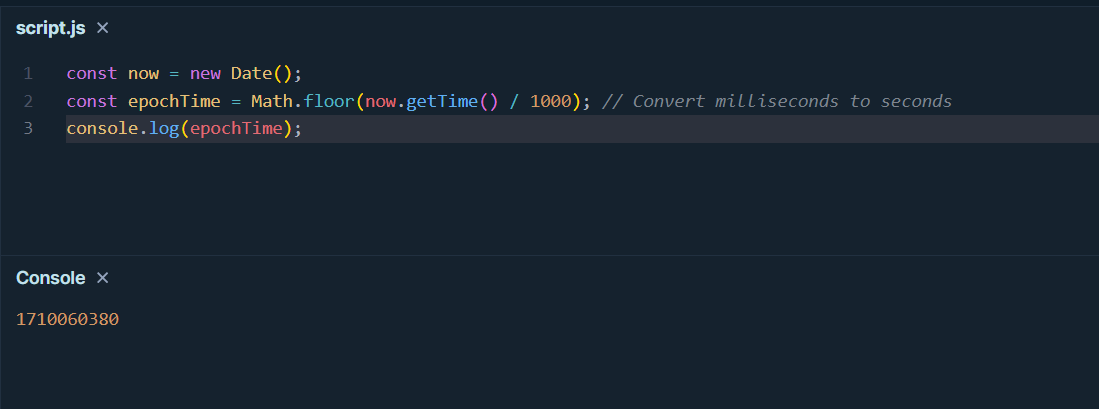
Learn more: