How to compare date sizes in JavaScript?
This article explains how to compare date and time in JavaScript. JavaScript offers various methods to represent date and time, including Date objects, timestamps, and date-time strings.
Handling dates is a very common and important task, whether it's scheduling meetings, devising plans, or tracking time in transactions. We often need to compare dates and times. This article will introduce how to compare dates and times in JavaScript, as well as tips and precautions in practical applications.
Date and time representation in JavaScript
JavaScript provides a Date object to handle dates and times. We can use it to create, manipulate, and represent dates and times.
// Create Date objects
let currentDate = new Date();
let futureDate = new Date('2024-12-31');
// Get date and time
console.log(currentDate); // Current date and time
console.log(futureDate); // Specified date and time
Date comparison methods in JavaScript
JavaScript provides multiple ways to represent dates and times, including Date objects, timestamps, and datetime strings. Details are as follows.
1 . Use comparison operators directly (>、<、>=、<=)
The Date object in JavaScript can be directly compared using comparison operators. When compared, the Date object is automatically converted to a millisecond value (the number of milliseconds since January 1, 1970).
const date1 = new Date('2024-03-17');
const date2 = new Date('2024-03-18');
if (date1 > date2) {
console.log('date1 is later than date2');
} else if (date1 < date2) {
console.log('date1 is earlier than date2');
} else {
console.log('The two dates are equal');
}
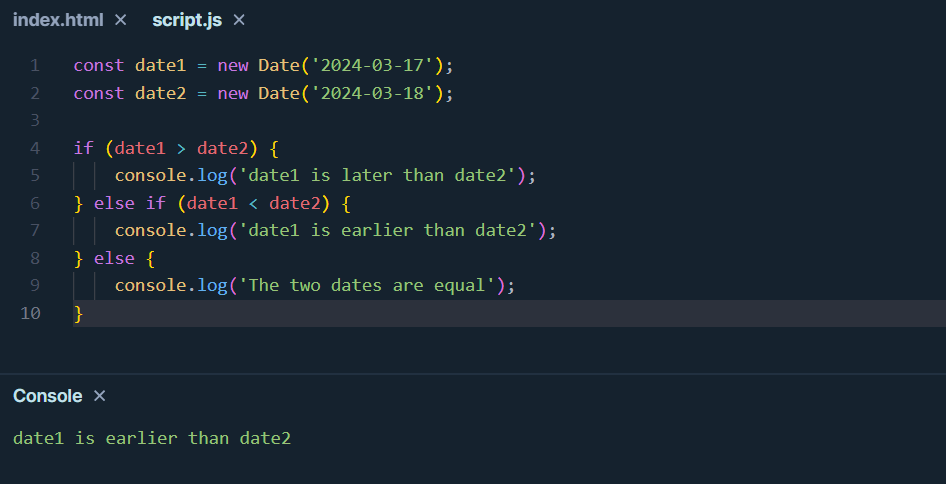
2. Compare date parts (ignore time)
If you only want to compare two dates without considering the time part, you can set the time part to the same value first and then compare them.
let date1 = new Date('2023-03-25T00:00:00');
let date2 = new Date('2023-03-26T00:00:00');
console.log(date1 < date2); // true
console.log(date1 >= date2); // false
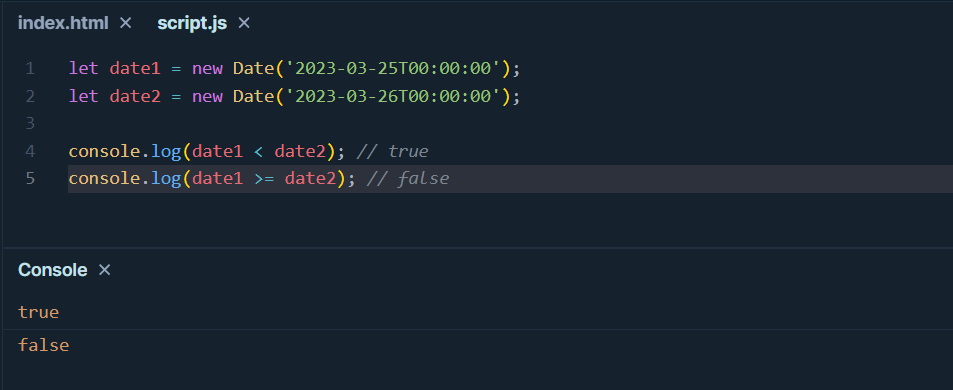
Alternatively, convert the time to a string in YYYY-MM-DD format and compare these strings:
let date1 = new Date('2023-03-25').toISOString().split('T')[0];
let date2 = new Date('2023-03-26').toISOString().split('T')[0];
console.log(date1 < date2); // true
console.log(date1 > date2); // false
3. Use Date.parse ()
Use the Date.parse ()
method to parse the date string, convert it to a millisecond value, and then compare.
let date1 = Date.parse('2023-03-25');
let date2 = Date.parse('2023-03-26');
console.log(date1 < date2); // true
console.log(date1 > date2); // false
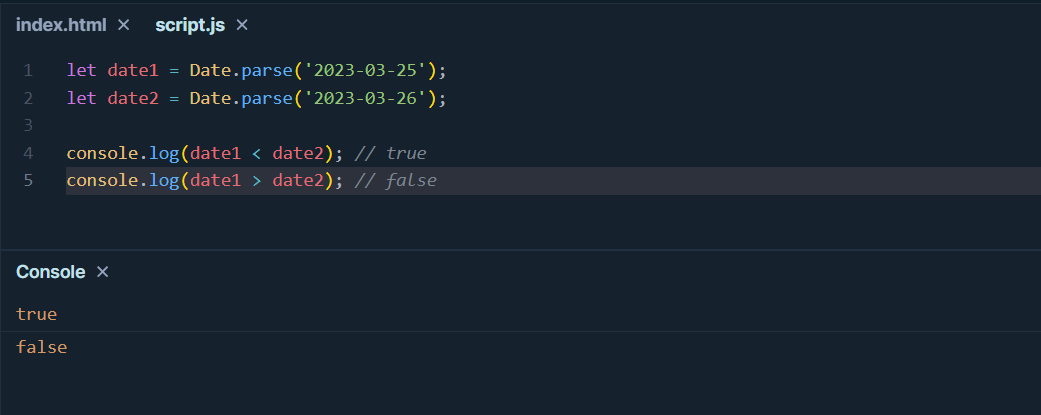
Comparative skills in practical application scenarios
In practical applications, we may need to compare the order of dates or check if they are equal.
// Compare the order of two dates
if (futureDate.getTime() > currentDate.getTime()) {
console.log('futureDate is later than currentDate');
} else if (futureDate.getTime() < currentDate.getTime()) {
console.log('futureDate is earlier than currentDate');
} else {
console.log('The two date times are equal');
}
// Check if two dates are equal
if (futureDate.getTime() === currentDate.getTime()) {
console.log('The two date times are equal');
} else {
console.log('The two date times are not equal');
}
Summary
This article introduces various methods for comparing dates and times, including using Date objects, timestamps, and datetime strings. Different methods are suitable for different scenarios, and developers can choose the appropriate method according to their needs. In the future, with the development of JavaScript technology, more convenient date and time processing tools and methods may emerge.
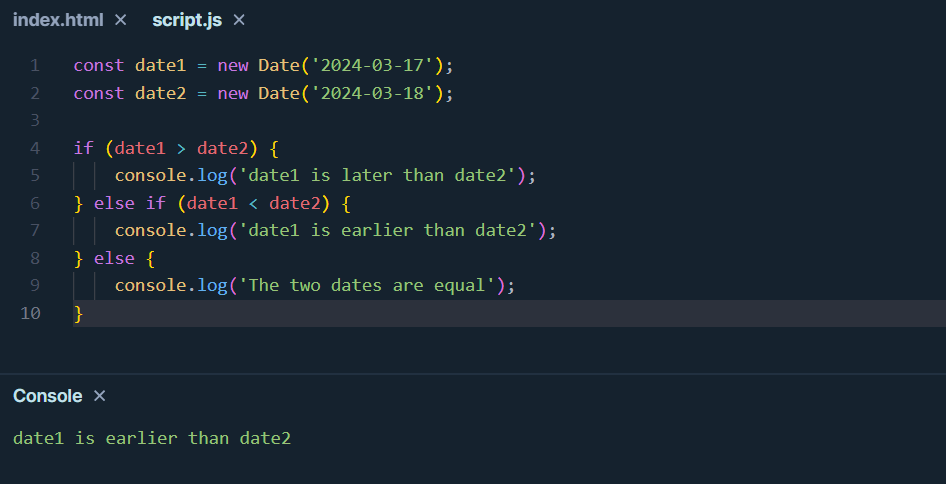
Learn more: