How to check if two arrays have equal values in JavaScript?
There are multiple methods to compare the values of two arrays in JavaScript, including loop comparison, using the toString() method of arrays, using JSON.stringify(), and utilizing ES6 features.
In JavaScript, there are often business needs to compare the values of two arrays to see if they are equal. This scenario may occur in various situations, such as validating user input or comparing data. This article will introduce several methods to introduce them.
Method 1: Cyclic comparison
First, we can use a loop to compare the elements of two arrays one by one. This method is very direct, just like comparing the values of two arrays one by one.
function areArraysEqual(array1, array2) {
if (array1.length !== array2.length) {
return false;
}
for (let i = 0; i < array1.length; i++) {
if (array1[i] !== array2[i]) {
return false;
}
}
return true;
}
const arr1 = [1, 2, 3];
const arr2 = [1, 2, 3];
console.log(areArraysEqual(arr1, arr2)); // Output: true
const arr3 = [1, 2, 3];
const arr4 = [1, 2, 4];
console.log(areArraysEqual(arr3, arr4)); // Output: false
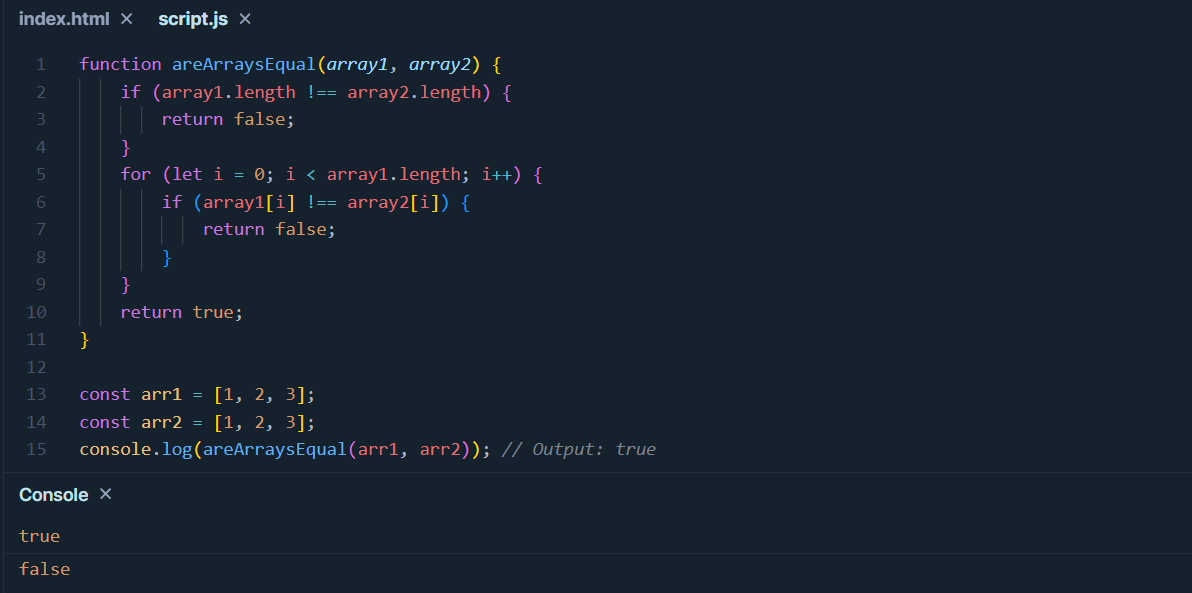
Method 2: Use the array's toString ()
method
Another way is to convert an array to a string and then compare the strings for equality. We can use the array's toString ()
method to achieve this conversion.
function areArraysEqual(array1, array2) {
return array1.toString() === array2.toString();
}
const arr1 = [1, 2, 3];
const arr2 = [1, 2, 3];
console.log(areArraysEqual(arr1, arr2)); // Output: true
const arr3 = [1, 2, 3];
const arr4 = [1, 2, 4];
console.log(areArraysEqual(arr3, arr4)); // Output: false
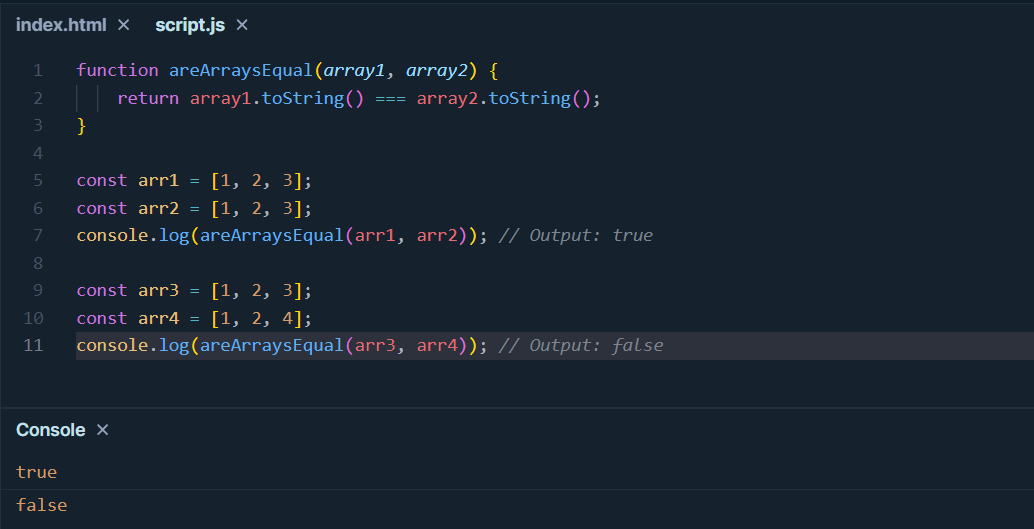
Method 3: Use JSON.stringify ()
We can also use the JSON.stringify ()
method to convert arrays to JSON strings and then compare them, which is relatively reliable and suitable for most situations.
function areArraysEqual(array1, array2) {
return JSON.stringify(array1) === JSON.stringify(array2);
}
const arr1 = [1, 2, 3];
const arr2 = [1, 2, 3];
console.log(areArraysEqual(arr1, arr2)); // Output: true
const arr3 = [1, 2, 3];
const arr4 = [1, 2, 4];
console.log(areArraysEqual(arr3, arr4)); // Output: false
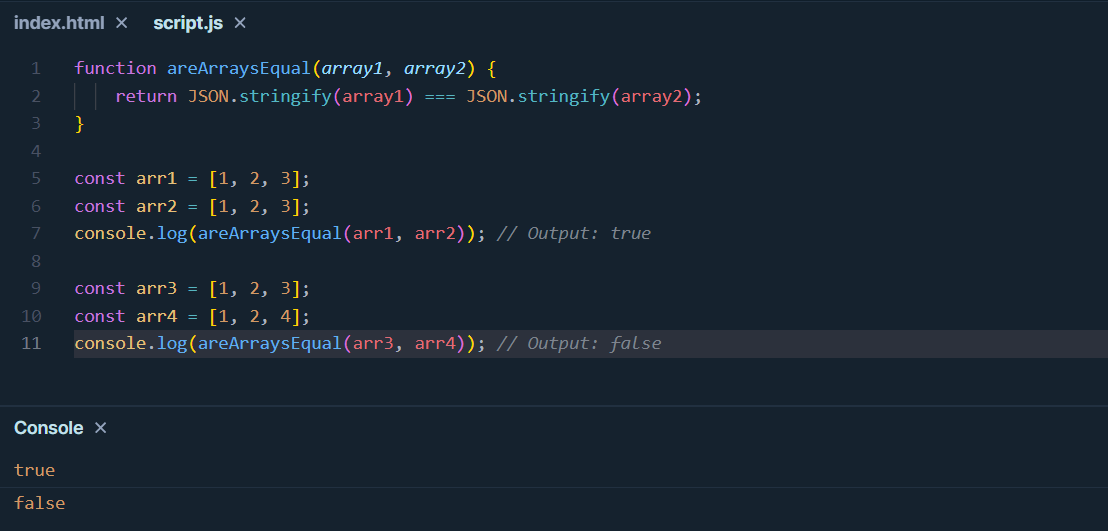
Method 4: Use the new ES6 features
In ES6, we can use some new features to simplify the array comparison code, such as Array.prototype.every ()
or Array.prototype.includes ()
.
function areArraysEqual(array1, array2) {
return array1.length === array2.length && array1.every((value, index) => value === array2[index]);
}
const arr1 = [1, 2, 3];
const arr2 = [1, 2, 3];
console.log(areArraysEqual(arr1, arr2)); // Output: true
const arr3 = [1, 2, 3];
const arr4 = [1, 2, 4];
console.log(areArraysEqual(arr3, arr4)); // Output: false
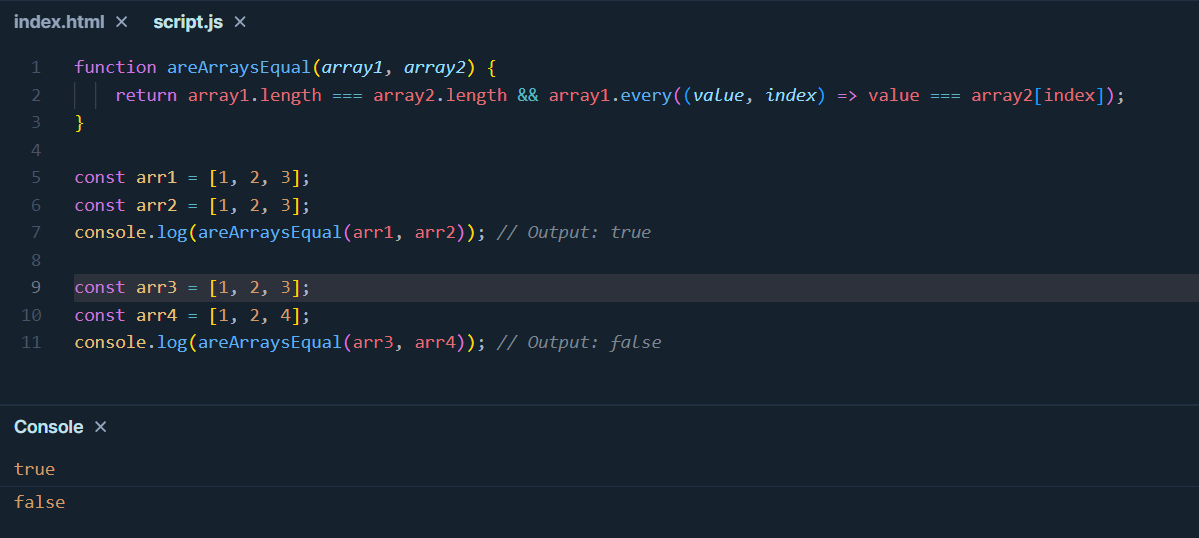
Summary
Overall, there are various ways to compare the values of two arrays in JavaScript. When choosing a method, various factors should be considered based on specific situations, such as performance, readability, and applicability. Different situations may require different methods to solve, so these methods should be flexibly used.
Reference link:
- MDN Documentation: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array
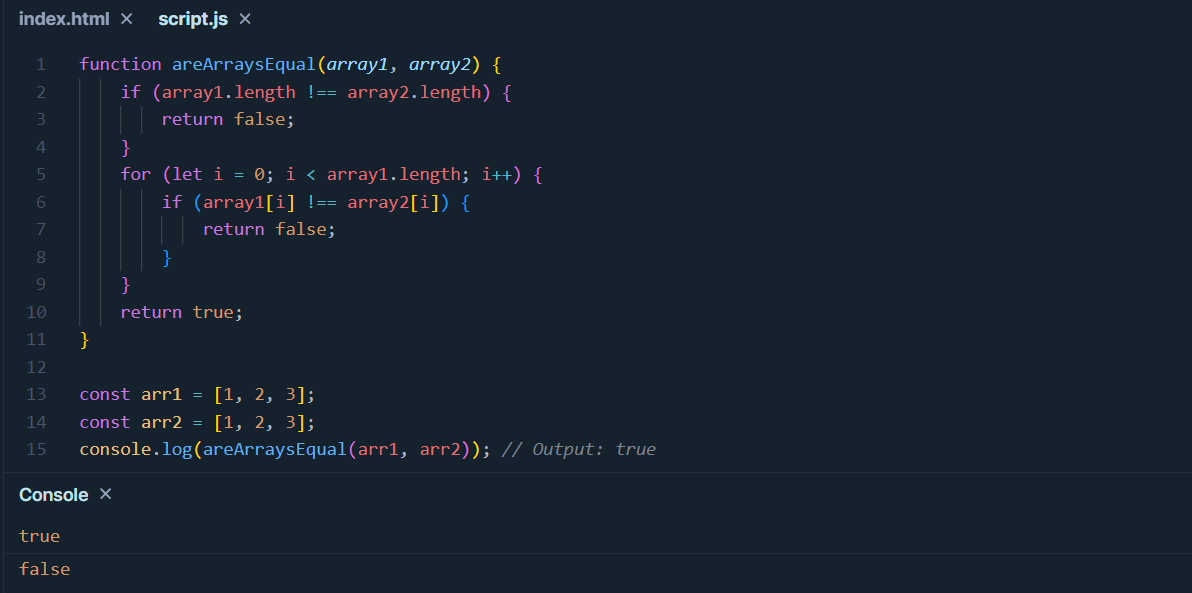
Learn more: