How to find the intersection of two arrays in JavaScript?
This article introduces several common methods for finding the intersection of two arrays in JavaScript, including using loops and conditional statements, array methods, the Set data structure in ES6, as well as the spread operator and filter method. You can easily achieve this goal.
When processing data, it is often necessary to find the intersection of two arrays, which can help us filter out common elements. JavaScript provides multiple methods to achieve this goal, and this article will introduce several common methods.
Method 1: Use loops and conditional statements
To find the intersection of two arrays, the most basic method can be used, which is to iterate through one array and search for the same elements in the other array. Although this method may seem cumbersome, it is very effective in practice. However, it should be noted that the efficiency of this method will be affected when the array is large.
function findIntersection(array1, array2) {
let intersection = [];
for (let i = 0; i < array1.length; i++) {
if (array2.indexOf(array1[i]) !== -1) {
intersection.push(array1[i]);
}
}
return intersection;
}
const array1 = [1, 2, 3, 4, 5];
const array2 = [4, 5, 6, 7, 8];
console.log(findIntersection(array1, array2)); // Output: [4, 5]
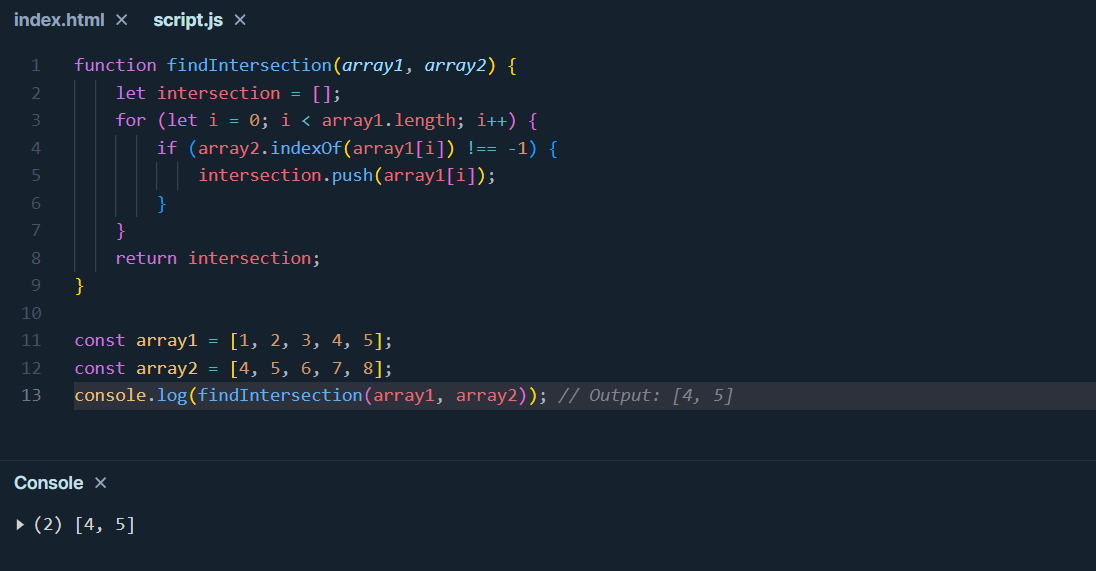
Method 2: Use array methods
JavaScript provides a series of powerful array methods, such as filter, includes, reduce, etc., which can make it easier for you to find the intersection of arrays. These methods can make your code more concise and readable.
function findIntersection(array1, array2) {
return array1.filter(element => array2.includes(element));
}
const array1 = [1, 2, 3, 4, 5];
const array2 = [4, 5, 6, 7, 8];
console.log(findIntersection(array1, array2)); // Output: [4, 5]
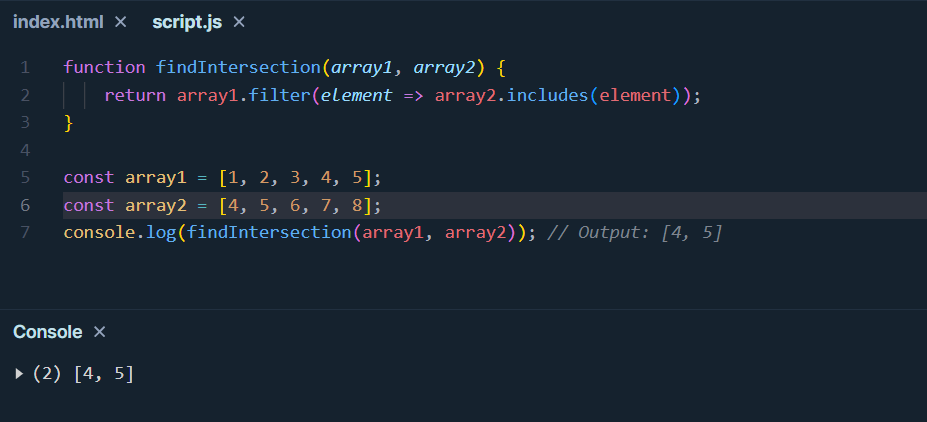
Method 3: Use the Set data structure in ES6
ES6 introduced the Set data structure, which is a collection that can automatically remove duplicate values. You can use the characteristics of Set to find the intersection of two arrays. However, it should be noted that Set can only handle unique values. If the array contains duplicate elements, extra attention should be paid.
function findIntersection(array1, array2) {
const set1 = new Set(array1);
const intersection = array2.filter(element => set1.has(element));
return intersection;
}
const array1 = [1, 2, 3, 4, 5];
const array2 = [4, 5, 6, 7, 8];
console.log(findIntersection(array1, array2)); // Output: [4, 5]
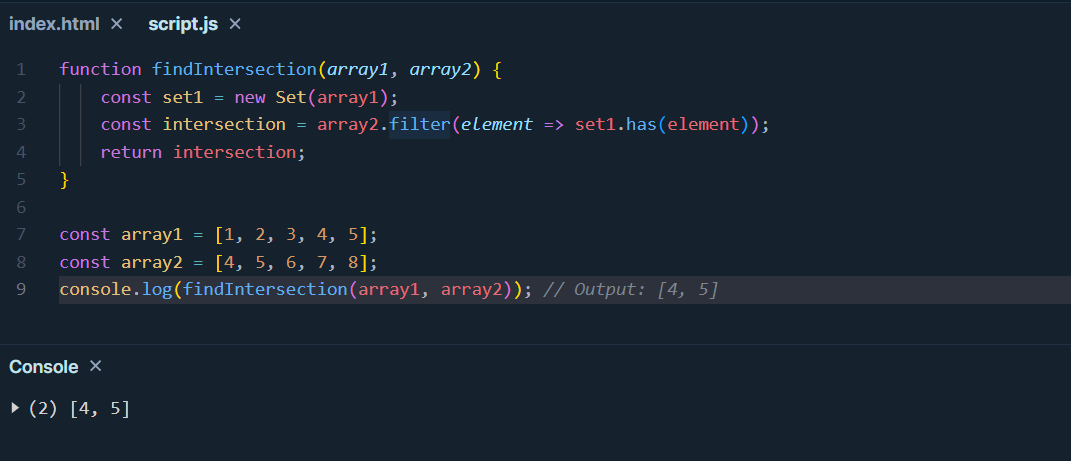
Method 4: Use the spread operator and filter method in ES6
The spread operator and filter method in ES6 are also a concise solution. You can convert an array to a Set and then use the filter method to find the intersection.
function findIntersection(array1, array2) {
const set1 = new Set(array1);
return array2.filter(element => set1.has(element));
}
const array1 = [1, 2, 3, 4, 5];
const array2 = [4, 5, 6, 7, 8];
console.log(findIntersection(array1, array2)); // Output: [4, 5]
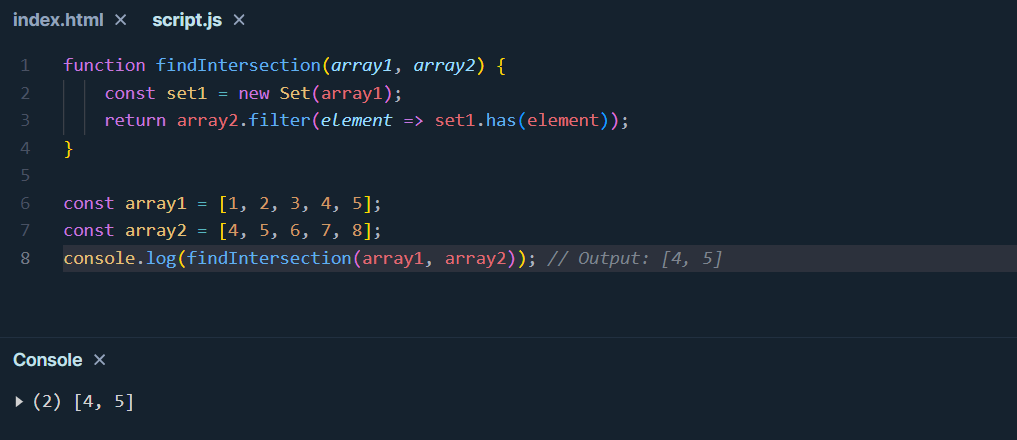
Summary
This article introduces several common methods for finding the intersection of two arrays in JavaScript, including using loops and conditional statements, array methods, the Set data structure in ES6, and extension operators and filter methods. You can easily achieve this goal.
Reference link:
- JavaScript MDN Documentation: https://developer.mozilla.org/en-US/docs/Web/JavaScript
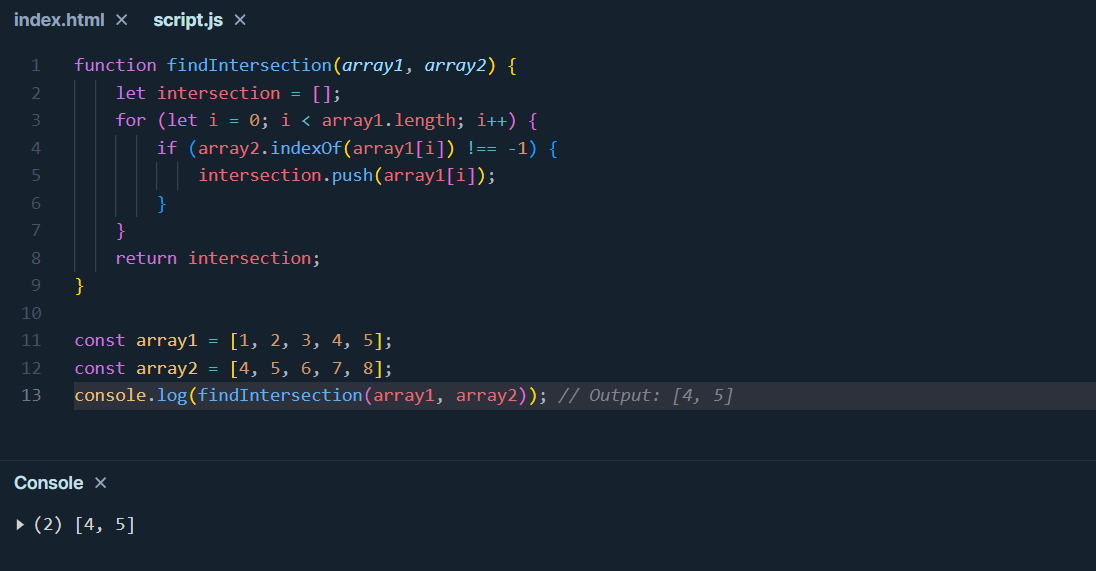
Learn more: