How to Add Minutes to Date in JavaScript?
How to add minutes and seconds to a date object in JavaScript? This article provides some convenient methods to handle this issue. We can use the methods of the Date object to add minutes and seconds, which are setMinutes() method and setSeconds() method respectively.
This article mainly discusses how to handle dates in JavaScript. In many projects, we need to perform some operations on dates, such as adding minutes and seconds to a date. These operations may involve timers, scheduled tasks, or simple time adjustments. Therefore, understanding how to add minutes and seconds to dates in JavaScript is very useful.
Date objects in JavaScript
First, let's understand the date object in JavaScript. JavaScript provides a built-in object called Date, which allows us to easily handle dates and times. We can use the new Date ()
to create a date object, or directly use ready-made methods to obtain the current date and time.
// Create a new date object
let currentDate = new Date();
// Get the current date and time
let currentDateTime = currentDate.toString();
console.log("Current date and time:", currentDateTime);
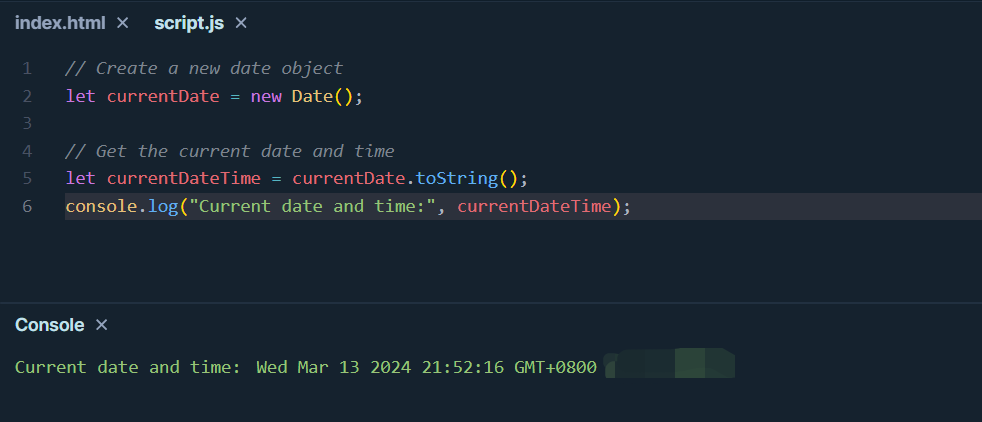
Add minutes and seconds to the date
Now, let's see how to add minutes and seconds to a date object in JavaScript. JavaScript provides some convenient methods to handle this problem. We can use the methods of the Date object to add minutes and seconds, which are the setMinutes()
method and the setSeconds()
method, as shown below. You can compare them by looking at the picture below, or run it yourself to see.
// Create a new date object
let currentDate = new Date();
// Get the current date and time
let currentDateTime = currentDate.toString();
console.log("Current date and time:", currentDateTime);
// Add minutes
currentDate.setMinutes(currentDate.getMinutes() + 10);
console.log("Date and time after adding 10 minutes:", currentDate.toString());
// Add seconds
currentDate.setSeconds(currentDate.getSeconds() + 30);
console.log("Date and time after adding 10 minutes and 30 seconds:", currentDate.toString());
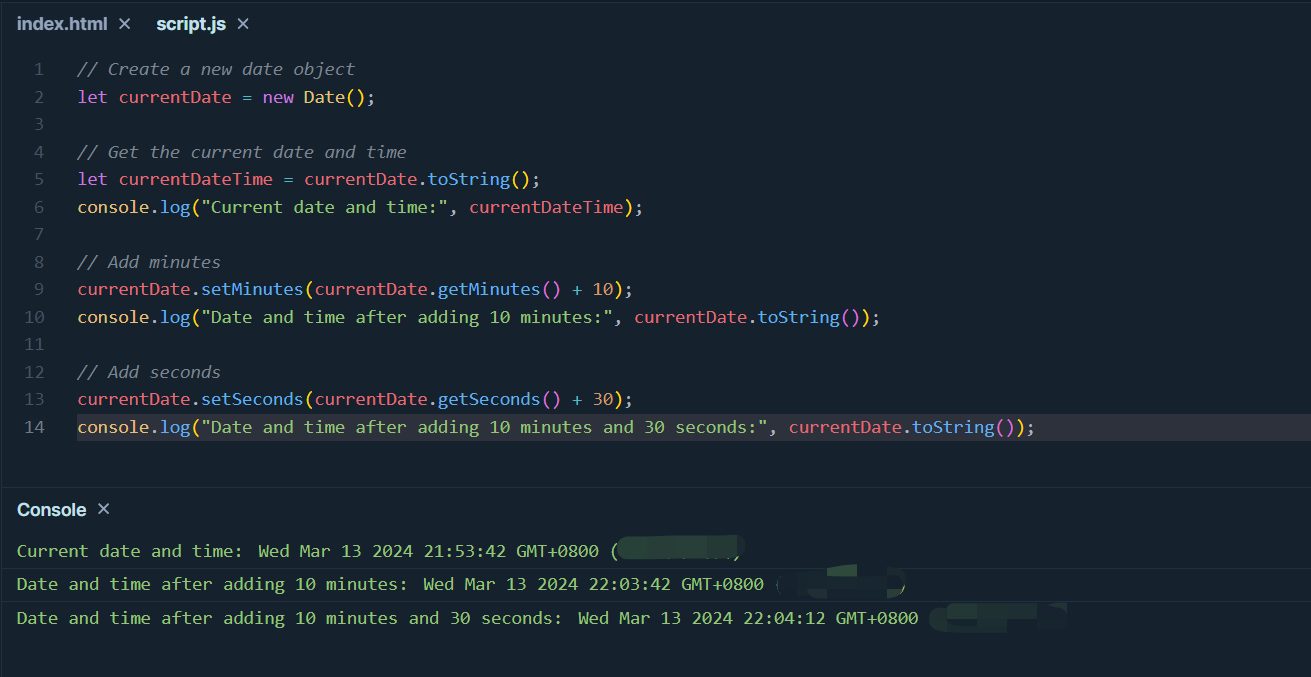
More examples
Let's look at a few examples to demonstrate how to add minutes and seconds to dates in practical applications.
// Example 1: Using in a Timer
let startDate = new Date();
console.log("Start time:", startDate.toString());
// Simulating a timer to execute after 10 minutes
let endDate = new Date(startDate);
endDate.setMinutes(endDate.getMinutes() + 10);
console.log("End time:", endDate.toString());
// Example 2: Using in Scheduled Task
let taskDate = new Date();
console.log("Task date:", taskDate.toString());
// Scheduled task will execute after 3 days
taskDate.setDate(taskDate.getDate() + 3);
console.log("Task execution date:", taskDate.toString());
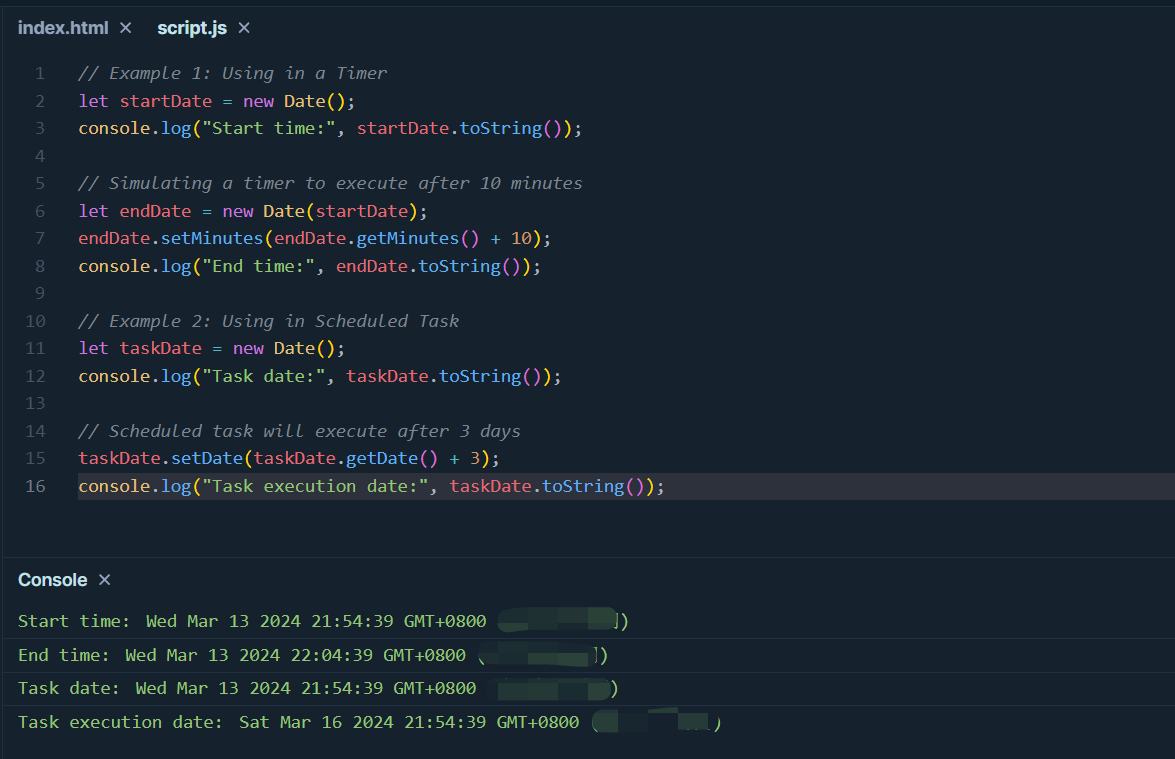
Notes
When dealing with date operations, make sure to consider the impact of time zones and daylight saving time, as well as avoid confusion and errors in date operations.
Summary
In general, adding minutes and seconds to dates in JavaScript is not complicated. By using the setMinutes()
and setSeconds()
methods of the Date object, we can easily achieve these operations.
Reference link:
- MDN Web Documentation: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date
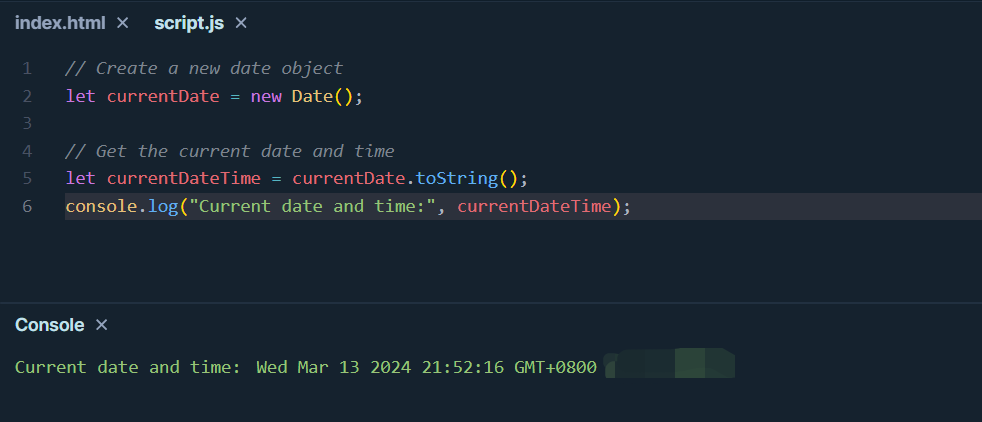
Learn more: