How to convert timestamps in Postman?
In Postman, timestamps can be converted using Moment.js library or native JS. Moment.js offers convenience, being built into Postman and providing the moment.unix(Number) method, while native JS code is slightly more cumbersome.
In Postman, handling timestamps is crucial for debugging and testing API requests and responses. Proper handling ensures data accuracy and consistency, with Moment.js library and native JS being two common approaches.
1.Converting Timestamps using Moment.js Library
Postman comes with the Moment.js library built-in, allowing conversion using the moment.unix(Number)
method. See the script below for reference:
let timestamp = Math.floor(Date.now() / 1000);
let moment = require("moment");
// Creating moment object directly from timestamp and formatting
let formattedDate = moment.unix(timestamp).format("YYYY-MM-DD HH:mm:ss");
console.log(formattedDate);
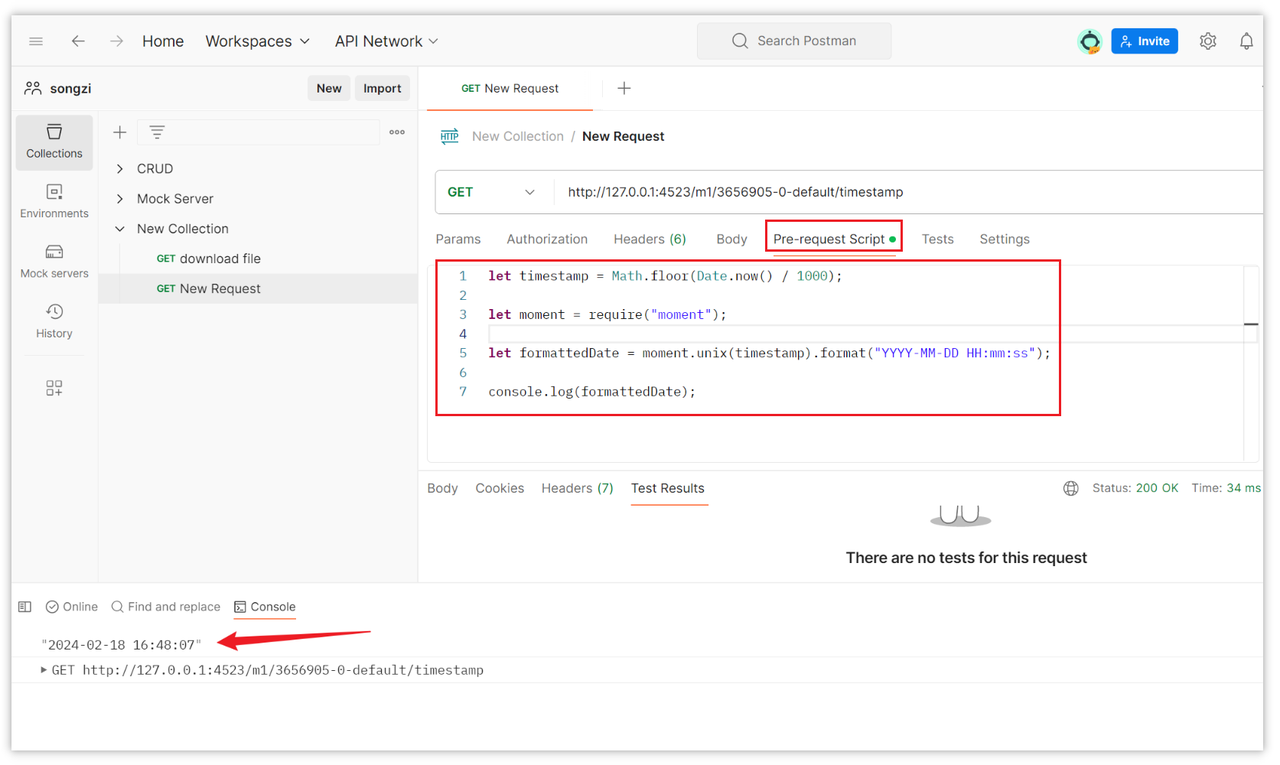
After conversion, you can add it to environment variables for later use. See the script below (added to the previous script):
// Adding timestamp to environment variables
pm.environment.set('current_timestamp', timestamp);
// Adding formatted date to request
pm.environment.set('current_date', formatDate(timestamp));
2.Converting Timestamps using Native JS
Converting timestamps using native JS requires more code and can be confusing, hence not recommended. See below for reference:
let timestamp = Math.floor(Date.now() / 1000);
function formatTimestamp(timestamp) {
const date = new Date(timestamp * 1000);
const year = date.getFullYear();
const month = (date.getMonth() + 1).toString().padStart(2, '0');
const day = date.getDate().toString().padStart(2, '0');
const hours = date.getHours().toString().padStart(2, '0');
const minutes = date.getMinutes().toString().padStart(2, '0');
const seconds = date.getSeconds().toString().padStart(2, '0');
const formattedDate = `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`;
return formattedDate;
}
const formattedDate = formatTimestamp(timestamp);
console.log(formattedDate);
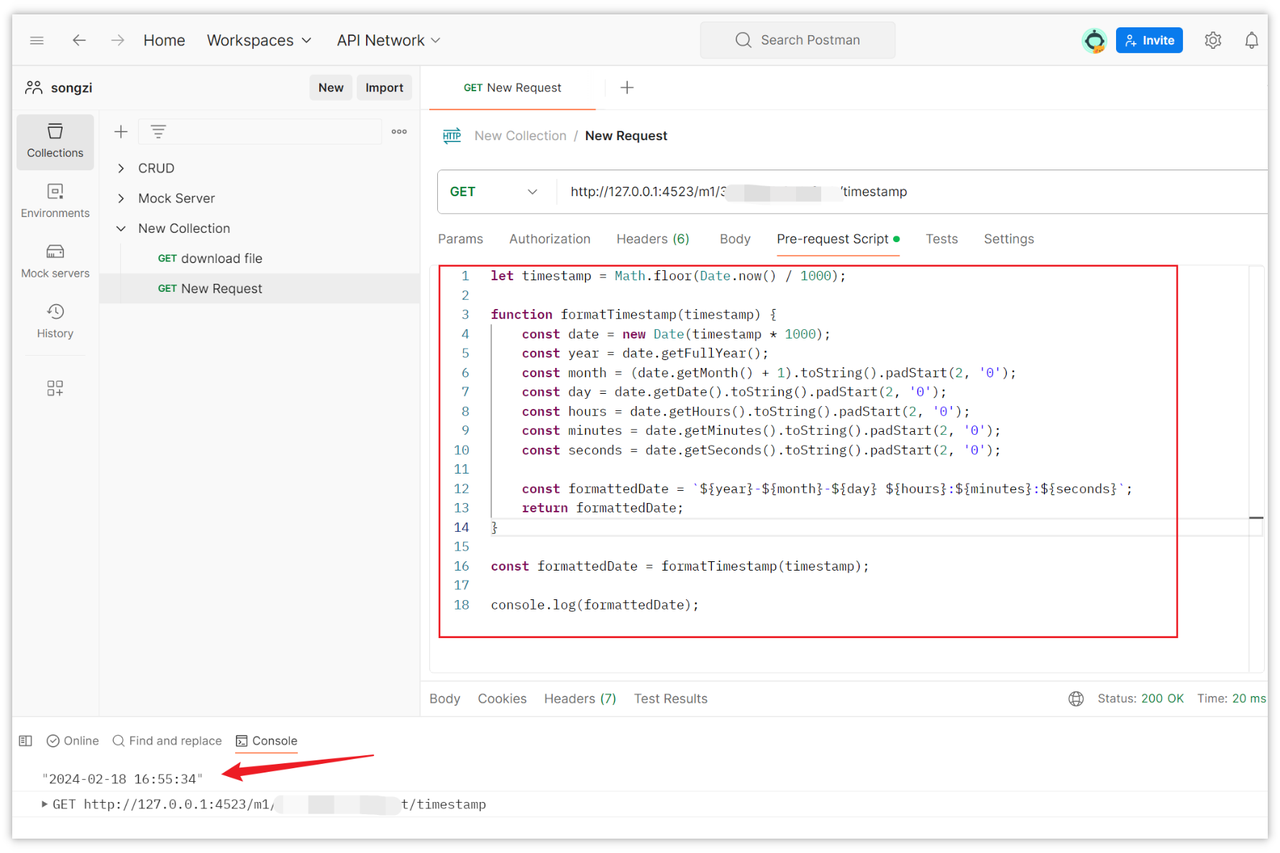
Conclusion
In Postman, timestamps can be converted using Moment.js library or native JS. Moment.js offers convenience, being built into Postman and providing the moment.unix(Number)
method, while native JS code is slightly more cumbersome.
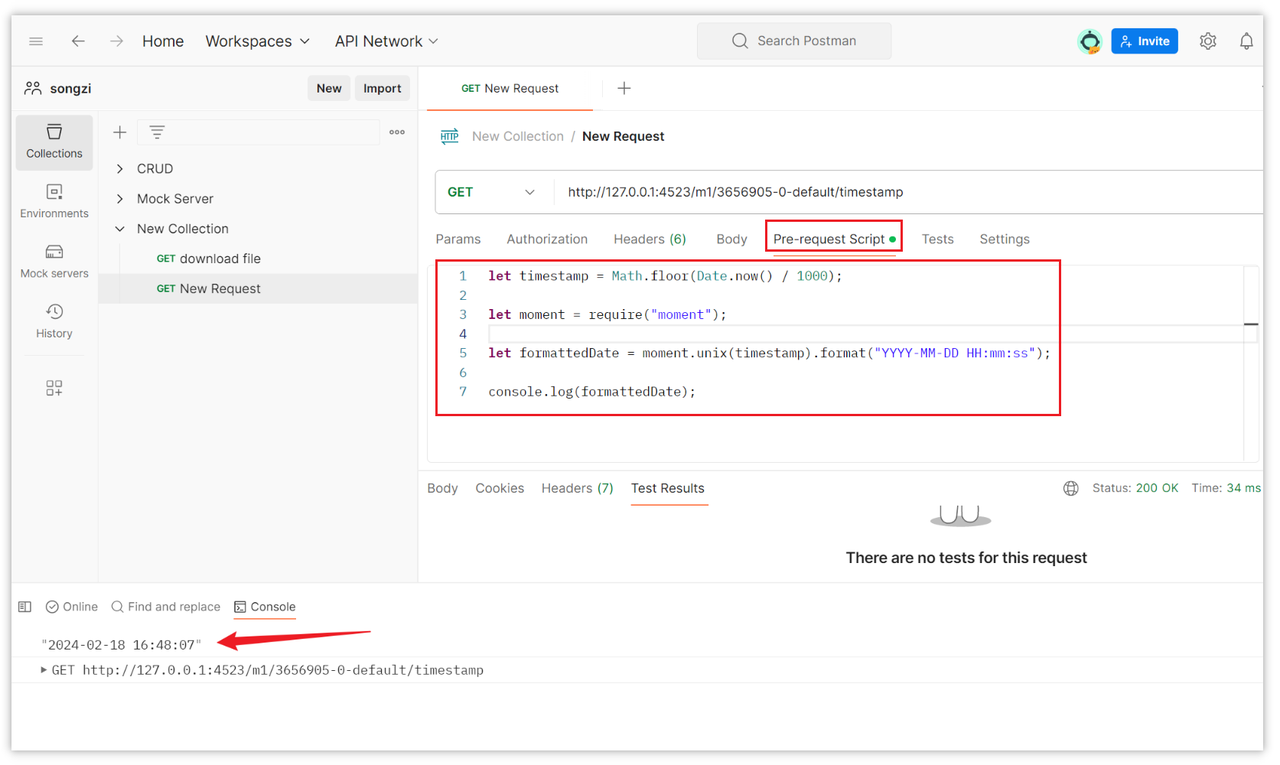
Learn more:
Learn more: